How to split the string in delphi
13,523
Solution 1
Create a TStringList
and assign your comma separated string to StringList.CommaText
. This parses your input and returns the split strings as the items of the string list.
StringList.CommaText := '"STANS", "Payment, chk# 1", ,1210.000';
//StringList[0]='STANS'
//StringList[1]='Payment, chk# 1'
//etc.
Solution 2
I wrote this function and works perfect for me in Delphi 2007
function ParseCSV(const S: string; ADelimiter: Char = ','; AQuoteChar: Char = '"'): TStrings;
type
TState = (sNone, sBegin, sEnd);
var
I: Integer;
state: TState;
token: string;
procedure AddToResult;
begin
if (token <> '') and (token[1] = AQuoteChar) then
begin
Delete(token, 1, 1);
Delete(token, Length(token), 1);
end;
Result.Add(token);
token := '';
end;
begin
Result := TstringList.Create;
state := sNone;
token := '';
I := 1;
while I <= Length(S) do
begin
case state of
sNone:
begin
if S[I] = ADelimiter then
begin
token := '';
AddToResult;
Inc(I);
Continue;
end;
state := sBegin;
end;
sBegin:
begin
if S[I] = ADelimiter then
if (token <> '') and (token[1] <> AQuoteChar) then
begin
state := sEnd;
Continue;
end;
if S[I] = AQuoteChar then
if (I = Length(S)) or (S[I + 1] = ADelimiter) then
state := sEnd;
end;
sEnd:
begin
state := sNone;
AddToResult;
Inc(I);
Continue;
end;
end;
token := token + S[I];
Inc(I);
end;
if token <> '' then
AddToResult;
if S[Length(S)] = ADelimiter then
AddToResult
end;
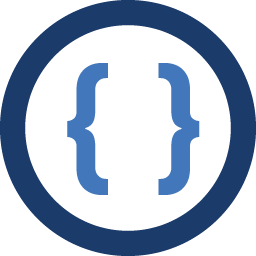
Author by
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
I just need to split a string like:
"STANS", "Payment, chk#1", ,1210.000
into an array based on,
. The result in the string list would beSTANS Payment, chk#1 1210.000
-
Alex almost 13 yearsIf it doesn't work quite right, there are also improved versions out on the net.
-
David Heffernan almost 13 years@mj2008 Could you elaborate please? This code works fine on the example given. If you are going to debunk an answer it is better to provide some evidence. If you have evidence then I will very happily stand corrected.
-
Alex almost 13 yearsI upvoted you, and think it is the correct answer. I wasn't out to "debunk" you! I have a TStringCSVList from Magenta Systems that does some space improvements, but it is from 2000 so maybe the current Delphi implementation is better. For good CSV, Delphi is fine out of the box. For dodgy CSV, there are similar things out there.
-
DearVolt almost 9 years@"David Heffernan" If the string contains spaces between the commas, but no double quotes, how would you go about this? For example, I have "900 024000649314,2015/07/04,08:23:12" as the first part of my line. If I do the following: ShowMessage(StringList[0]); It outputs only 900. Thanks in advance!
-
David Heffernan almost 9 years@DJSquared Set
StrictDelimiter
toTrue
. -
DearVolt almost 9 yearsDelphi 7 doesn't support the above mentioned, so I'm going to use on of the tricks mentioned on this thread: stackoverflow.com/questions/1335027/… Thank you!
-
David Heffernan almost 9 years@DJSquared If I were you I would use a bespoke split function. How about my one from here: stackoverflow.com/questions/28410901/…