how to start a new activity after login success?
13,122
Solution 1
You can put intent in your onPostExecute method like this :
@Override
protected void onPostExecute(String result) {
if(result == null)
{
// do what you want to do
}
else if(result.equals("Registration Success..."))
{
Toast.makeText(ctx, result, Toast.LENGTH_LONG).show();
}
else if(result.contains("Login Success")) // msg you get from success like "Login Success"
{
Intent i = new Intent(ctx,NewActivity.class);
ctx.startActivity(i);
alertDialog.setMessage(result);
alertDialog.show();
}
}
Edit : If you want to call new activity after login success then put intent in else part.
Solution 2
try this
Intent intent = new Intent(MainActivity.this, Second.class);
startActivity(intent);
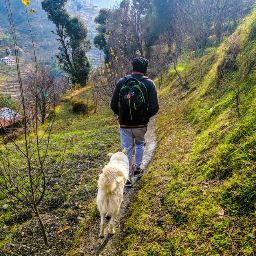
Author by
rijo raju
Updated on June 07, 2022Comments
-
rijo raju almost 2 years
i want to start a new activity after login success. that is when login goes correct a new activity should start automatically...i dont know where to mention startactivity. please help me with this, im a newbie to android. this is my code...
public class BackgroundTask extends AsyncTask<String,Void,String> { AlertDialog alertDialog; Context ctx; BackgroundTask(Context ctx) { this.ctx =ctx; } @Override protected void onPreExecute() { alertDialog = new AlertDialog.Builder(ctx).create(); alertDialog.setTitle("Login Information...."); } @Override protected String doInBackground(String... params) { String reg_url = ""; String login_url = ""; String method = params[0]; if (method.equals("register")) { String name = params[1]; String user_name = params[2]; String user_pass = params[3]; try { URL url = new URL(reg_url); HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection(); httpURLConnection.setRequestMethod("POST"); httpURLConnection.setDoOutput(true); //httpURLConnection.setDoInput(true); OutputStream OS = httpURLConnection.getOutputStream(); BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(OS, "UTF-8")); String data = URLEncoder.encode("user", "UTF-8") + "=" + URLEncoder.encode(name, "UTF-8") + "&" + URLEncoder.encode("user_name", "UTF-8") + "=" + URLEncoder.encode(user_name, "UTF-8") + "&" + URLEncoder.encode("user_pass", "UTF-8") + "=" + URLEncoder.encode(user_pass, "UTF-8"); bufferedWriter.write(data); bufferedWriter.flush(); bufferedWriter.close(); OS.close(); InputStream IS = httpURLConnection.getInputStream(); IS.close(); //httpURLConnection.connect(); httpURLConnection.disconnect(); return "Registration Success..."; } catch (MalformedURLException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } else if(method.equals("login")) { String login_name = params[1]; String login_pass = params[2]; try { URL url = new URL(login_url); HttpURLConnection httpURLConnection = (HttpURLConnection)url.openConnection(); httpURLConnection.setRequestMethod("POST"); httpURLConnection.setDoOutput(true); httpURLConnection.setDoInput(true); OutputStream outputStream = httpURLConnection.getOutputStream(); BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(outputStream,"UTF-8")); String data = URLEncoder.encode("login_name","UTF-8")+"="+URLEncoder.encode(login_name,"UTF-8")+"&"+ URLEncoder.encode("login_pass","UTF-8")+"="+URLEncoder.encode(login_pass,"UTF-8"); bufferedWriter.write(data); bufferedWriter.flush(); bufferedWriter.close(); outputStream.close(); InputStream inputStream = httpURLConnection.getInputStream(); BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream,"iso-8859-1")); String response = ""; String line = ""; while ((line = bufferedReader.readLine())!=null) { response+= line; } bufferedReader.close(); inputStream.close(); httpURLConnection.disconnect(); return response; } catch (MalformedURLException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } return null; } @Override protected void onProgressUpdate(Void... values) { super.onProgressUpdate(values); } @Override protected void onPostExecute(String result) { if(result.equals("Registration Success...")) { Toast.makeText(ctx, result, Toast.LENGTH_LONG).show(); } else { alertDialog.setMessage(result); alertDialog.show(); } } }
-
rijo raju over 8 yearsthanks for the reply. but if i put intent here then it will execute after registration success...right? but i want it after login success
-
SANAT over 8 years@rijoraju it helps you?
-
rijo raju over 8 yearsbuddy im getting some error...cannot resolve startactivity method
-
SANAT over 8 years@rijoraju use context.startActivity(i);
-
rijo raju over 8 yearsIntent i = new Intent(BackgroundTask.this,Main2Activity.class); BackgroundTask.startActivity(i); this is what i did..but it still gives me error.. sorry im just a noob
-
SANAT over 8 years@rijoraju use ctx.startActivity(i);
-
user5292387 over 8 yearsUnless your asynctask has a reference to a Context, you'll get the unresolved error for startActivity.
-
SANAT over 8 years@rijoraju check edited answer based on your variable used.
-
SANAT over 8 years@rijoraju it helps you?
-
rijo raju over 8 yearsthanks sanat shukla...it worked, really thanks. and one more thing...can i delete this @Override protected void onPreExecute() { alertDialog = new AlertDialog.Builder(ctx).create(); alertDialog.setTitle("Login Information...."); }
-
SANAT over 8 years@rijoraju if you don't want alert dialog then delete it.
-
user5292387 over 8 yearsUpvoted. I have no idea why someone would downvote an answer that 1) solves the problem and 2) shows that the person read all the code provided. Unless of course the downvoter didn't bother to read everything.
-
Pavan over 8 years@sanatshukla just one question what if registration fails and also login fails?
-
rijo raju over 8 yearseven after login fails...it still going to the next activity..this is not what i wanted..i wanted the app to move to next activity only when login success
-
rijo raju over 8 years@sanatshukla not working now even without fillling the login details if i click on login the new activity starts
-
Sagar Zala over 8 yearsYou have to declare method variable global in the BackgroundTask class.
-
rijo raju over 8 yearssorry buddy im still getting error could you just declare it please its a request
-
SANAT over 8 years@rijoraju sorry for late reply its my fault. I edited my answer. First need to check for null and then other conditions.
-
rijo raju over 8 yearsstill the same result..without any login details it moves to new activity on click
-
SANAT over 8 years@rijoraju what u get in login success ?
-
Sagar Zala over 8 yearsdid you added this sentence- method = params[0];
-
Sagar Zala over 8 yearsPlease see my answer.
-
rijo raju over 8 yearswithout applying your code..i get a alert saying login success...welcome rijo, but after applying your code even without entering any detail login it moves to new activity
-
SANAT over 8 years@rijoraju check my edited answer. Put your exact msg value to else if condition.
-
rijo raju over 8 yearsi tried with your code...but it not starting a new activity on login success..its only giving the alert that is login success
-
rijo raju over 8 yearsthe exact msg i get is ....Login Success...Welcome Rijo Raju where Rijo Raju is retrieved from the database but i dont know about welcome.
-
SANAT over 8 yearsUse status code from server side for easiness. Otherwise it becomes complicated.
-
rijo raju over 8 yearsstill im not able to do it...i feel like this app not gonna work
-
SANAT over 8 years@rijoraju Implementation is not good for the web service. Web service always return something like status code (200,400 etc) based on which you can identify what you want to do next in your app. For last time i m doing something in answer check edited answer after some time
-
SANAT over 8 years@rijoraju answer edited , now give it a last chance.
-
rijo raju over 8 yearsbuddy can you help with something more.
-
rijo raju over 8 yearsbuddy i want to show all the data from the mysql database on my app...i tried different method..not working for me...please help me with this i tried this method geeks.gallery/…