How to store complex object in redis hash in c#?
Solution 1
Probably the easiest path is checking if each property value is a collection (see the comments in my modified version of your method):
public static HashEntry[] ToHashEntries(this object obj)
{
PropertyInfo[] properties = obj.GetType().GetProperties();
return properties
.Where(x => x.GetValue(obj) != null) // <-- PREVENT NullReferenceException
.Select
(
property =>
{
object propertyValue = property.GetValue(obj);
string hashValue;
// This will detect if given property value is
// enumerable, which is a good reason to serialize it
// as JSON!
if(propertyValue is IEnumerable<object>)
{
// So you use JSON.NET to serialize the property
// value as JSON
hashValue = JsonConvert.SerializeObject(propertyValue);
}
else
{
hashValue = propertyValue.ToString();
}
return new HashEntry(property.Name, hashValue);
}
)
.ToArray();
}
Solution 2
It seems that there is something wrong with serializing. The best way of converting between JSON and .NET object is using the JsonSerializer:
JsonConvert.SerializeObject(fooObject);
You can see more details from Serializing and Deserializing JSON.
Also there is another good way,you can try to use IRedisTypedClient
which is a part of ServiceStack.Redis.
IRedisTypedClient - A high-level 'strongly-typed' API available on Service Stack's C# Redis Client to make all Redis Value operations to apply against any c# type. Where all complex types are transparently serialized to JSON using ServiceStack JsonSerializer - The fastest JSON Serializer for .NET.
Hope this helps.
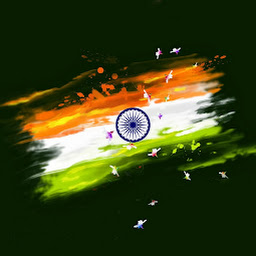
dharmesh parmar
Updated on June 04, 2022Comments
-
dharmesh parmar almost 2 years
I have to store complex object into hash of redis cash.I am using stackexchange.redis to do this.My Class is like below.
public class Company { public string CompanyName { get; set; } public List<User> UserList { get; set; } } public class User { public string Firstname { get; set; } public string Lastname { get; set; } public string Twitter { get; set; } public string Blog { get; set; } }
My code snippet to store data in redis is:
db.HashSet("Red:10000",comapny.ToHashEntries());
//Serialize in Redis format:
public static HashEntry[] ToHashEntries(this object obj) { PropertyInfo[] properties = obj.GetType().GetProperties(); return properties .Where(x => x.GetValue(obj) != null) // <-- PREVENT NullReferenceException .Select(property => new HashEntry(property.Name, property.GetValue(obj) .ToString())).ToArray(); }
I could store the data in redis but not as i want.I am geting result as shown in below image.
I want
UserList
value in json format.So,how can i do this.