How to store object in mongoose schema?
12,988
Solution 1
You must create another mongoose schema:
var address = Schema({ street: String, city: String});
And the type of addresses will be Array< address >
Solution 2
You are actually storing an array.
var personSchema = Schema({
_id : Number,
name : String,
surname : String,
addresses : []
});
Solution 3
The Solution to save array is much simple,Please check below code
Adv.save({addresses: JSON.stringify(addresses)})
Your schema will look like it
var personSchema = Schema({
_id : Number,
name : String,
surname : String,
addresses : String,
});
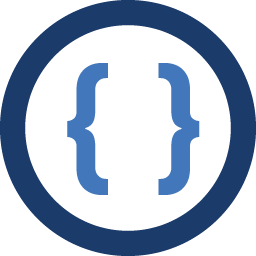
Author by
Admin
Updated on June 10, 2022Comments
-
Admin almost 2 years
In node.js I have three variables:
var name = 'Peter'; var surname = 'Bloom'; var addresses = [ {street: 'W Division', city: 'Chicago'}, {street: 'Beekman', city: 'New York'}, {street: 'Florence', city: 'Los Angeles'}, ];
And schema:
var mongoose = require('mongoose') , Schema = mongoose.Schema; var personSchema = Schema({ _id : Number, name : String, surname : String, addresses : ???? });
What type and how do I use it in schema? How is the best way for this?