How to store standard composition Classes in ObjectBox?
If you're saying Class A
contains a List of Class B
, then their relationship isn't a subclass/superclass but it's a standard composition. I understand your definition looks somewhat like this:
class A {
List<B> bs;
}
class B {
List<C> cs;
}
class C {}
And in order to use it in ObjectBox, you can define them as relations, either a standalone ToMany
relation, or a ToOne
relation with a backlink - depends on what better describes your data, you can achieve the same with both.
1st alternative - using ToMany
ToMany
relation stores the info in a separate "table" of IDs, like: A.id <--> B.id
and another one between B.id <--> C.id
.
@Entity()
class A {
int id;
final bs = ToMany<B>();
}
@Entity()
class B {
int id;
final cs = ToMany<C>;
}
@Entity()
class C {
int id;
}
2nd alternative - using ToOne with a backlink
ToOne
relation can achieve the same in your case and the relatin info is actually stored on the object itself as a single ID, i.e. there's a hidden int B.classAId
field that and you can get all Bs
for a class A
instance by looking for those Bs
pointing to this A
. This is done behind the scenes by a ToMany<>
with a Backlink()
annotation.
@Entity()
class A {
int id;
@Backlink()
final bs = ToMany<B>();
}
@Entity()
class B {
int id;
final a = ToOne<A>;
@Backlink()
final cs = ToMany<C>;
}
@Entity()
class C {
int id;
final b = ToOne<B>;
}
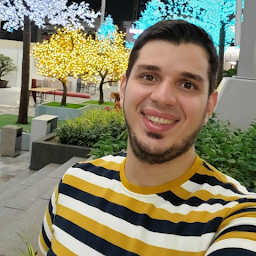
Baraa Aljabban
Updated on November 25, 2022Comments
-
Baraa Aljabban over 1 year
I have Class A contains List of Class B that contains List of Class C so how I would achieve this in ObjectBox ? how do I store it?
in the pic below you can see that I have
BasicInfo Class
ContainsData class
and so on....I put @Entinty annotation on
BasicInfo class
and after generating the modelsclass D
doesn't show up in theobjectbox.g.dart
file as it's on of BasicInfo propertiesModelEntity( id: const IdUid(1, 3501283007979667013), name: 'BasicInfo', lastPropertyId: const IdUid(1, 2073579066569957521), flags: 0, properties: <ModelProperty>[ ModelProperty( id: const IdUid(1, 2073579066569957521), name: 'id', type: 6, flags: 1) ], relations: <ModelRelation>[], backlinks: <ModelBacklink>[])
-
Baraa Aljabban almost 3 yearsThanks Vaind! yes you are correct about that, and it's my bad to call it subclass, I'll fix it now. So in my case there will be a for loop inside one another, to store the composite classes, and take the Id of first class and pass it as Target to the other class, right?
-
vaind almost 3 yearshard to tell what exactly you mean without a bit of code but it sounds reasonable :)