How to store string values in context.xml
Solution 1
You can configure named values that will be made visible to the web application as servlet context initialization parameters by nesting elements inside this element. For example, you can create an initialization parameter like this:
<Context>
...
<Parameter name="companyName" value="My Company, Incorporated"
override="false"/>
...
</Context>
This is equivalent to the inclusion of the following element in the web application deployment descriptor (/WEB-INF/web.xml):
<context-param>
<param-name>companyName</param-name>
<param-value>My Company, Incorporated</param-value>
</context-param>
Your java code looks like this
ServletContext sc = getServletContext();
String companyName = sc.getInitParameter("companyName");
Please see the reference http://tomcat.apache.org/tomcat-7.0-doc/config/context.html
Solution 2
You can use an Environment
tag:
<Context>
<Environment name="myConnectionURL" value="amqp:5272//blah.example.com¶m1=4" type="java.lang.String"/>
</Context>
And you can read it almost as you specified in the question:
InitialContext initialContext = new InitialContext();
Context environmentContext = (Context) initialContext.lookup("java:/comp/env");
String connectionURL = (String) environmentContext.lookup("myConnectionURL");
This is much the same as using a Parameter
tag, but without the need for a ServletContext
.
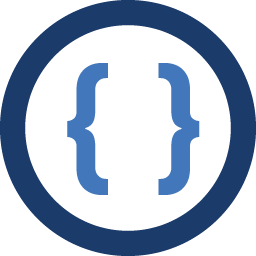
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I'd like to store connection URLs in a JNDI binding for my Tomcat application. Since Tomcat uses
context.xml
for JNDI resource defining, I need to figure out the propert way to store a String (or multiple strings for multiple connections) incontext.xml
.My reason for doing this is so that I can define different strings for different environments, and load them through JNDI.
Usually, I see entries like so:
<Context ...> <Resource name="someName" auth="Container" type="someFullyQualifiedClassName" description="Some description."/> </Context>
Is it really just as simple as:
<Context ...> <Resource name="myConnectionURL" auth="Container" type="java.lang.String" description="A connection URL string."/> </Context>
If so, where do I actually store the String value?!?! And if it's not correct, then what is the proper way for me to store, for instance, "
amqp:5272//blah.example.com¶m1=4
" incontext.xml
so I could then look it up like so:Context ctx = new InitialContext(); String connectionURL = (String)ctx.lookup("myConnectionURL");
Thanks in advance!
-
loesak about 9 yearsthis does not answer the question asked even though it is marked as the answer and may have helped the original question submitter. For those of you coming to this post looking for a solution for the posted question, refer to the answer provided by @Stoffe
-
gkiko almost 9 yearsShould the file name be
context.xml
and where is it stored? -
Stoffe almost 9 years@gkiko: in Tomcat it's called
context.xml
and there should already be one in theconf
directory of your tomcat installation, on *nix usually at/usr/share/tomcat/conf/context.xml
. Other web servers have other files and conventions.