How to style an unordered list with jQuery UI so that the elements begin with a UI icon instead of the default list symbol?
Solution 1
I know this question is a little out-of-date - but here is a fully working example:
HTML:
<div>
<ul class="icon person">
<li>John Doe</li>
<li>Jack Snow</li>
<li>Mary Moe</li>
</ul>
</div>
CSS:
ul.icon {
list-style: none; /* This removes the default bullets */
padding-left: 20px; /* This provides proper indentation for your icons */
}
ul.icon li {
position: relative; /* Allows you to absolutely place the :before element
relative to the <li>'s bounding box. */
}
ul.icon.person li:before {
background: url(/images/ui-icons_888888_256x240.png) -144px -96px;
/* ex: download.jqueryui.com/themeroller/images/ui-icons_888888_256x240.png */
/* The -144px, -96px coordinate is the location of the 16x16 Person icon */
/* The next 2 lines are necessary in order to make the :before pseudo-element
appear, and thereby show it's background, your icon. */
content: '';
display: inline-block;
/* Absolute is always in relation to the nearest positioned parent. In this
case, that's the <li> with _relative_ positioning, above. */
position: absolute;
left: -16px; /* Places the icon 16px left of the <li>'s edge */
top: 2px; /* Adjust this based on your font-size and line-height */
height: 16px; width: 16px; /* jQuery UI icons (with spacing) are 16x16 */
}
Here is a jsFiddle showing it working. The result will look something like this:
The reason that I used the :before
pseudo-element is that you are wanting to use jQuery-UI icons - which come in the form of a sprite map. In other words - all of the icons are found in a grid pattern within a single image, as in this example:
Source: http://download.jqueryui.com/themeroller/images/ui-icons_888888_256x240.png
If you tried to just make the background of the <li>
that image, it would be more complicated to make the single icon that you want appear without also displaying all of its neighbors. By using the :before
element, though, you can create a smaller, 16px
by 16px
box for the icon, and thereby easily trim down to showing only one icon.
If you want to learn more about :before
and :after
pseudo-elements, check out this fantastic article as a starting point.
Solution 2
Looking at the source for the page, just set the class file of the list item to clear the standard element, and then set a span tag to add the new icon.
<li class="ui-state-default ui-corner-all">
<span class="ui-icon ui-icon-person"></span>
</li>
Solution 3
ul li {
list-style-type: none;
background: url('your/path/image.png') no-repeat left center;
}
You may need to add some left padding as well.
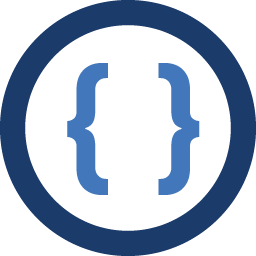
Admin
Updated on July 25, 2022Comments
-
Admin almost 2 years
I would like to use an icon from the jQuery UI icon set to style an unordered list.
<div> <ul> <li>John Doe</li> <li>Jack Snow</li> <li>Mary Moe</li> </ul> </div>
By default this list appears with dots in front of each element:
- John Doe
- Jack Snow
- Mary Moe
Instead I would like to replace the dot with an icon such as
ui-icon-person
How to accomplish this?