how to throw HTTP 204 status code using jersey framework in RESTful web service?
Solution 1
First, 204 is in the "Successful" category of response codes, so returning it as the result of an exception is a very, very weird thing to do.
Second, 204 means "No Content", meaning that the response contains no entity, but you put one in it. It's likely that Jersey is switching it to a 200 for you, which is basically identical to a 204 except that it contains a response entity.
Finally, you can get 204 responses very simply by a couple of built-in behaviors: void methods and null return values both map to a 204 response. Otherwise, simply return Response.status(204).build()
.
Solution 2
You shouldn't give entity if you want throw 204:
@GET
@Produces(MediaType.TEXT_PLAIN)
public Response test() {
//return Response.status(Status.NO_CONTENT).entity("hello").build(); //this will throw 200
return Response.status(Status.NO_CONTENT).build();
}
Solution 3
Just one thing to add to the already existing responses. What Jersey is doing is the correct behavior as for the spec:
3.3.3 Return Type
Resource methods MAY return void, Response, GenericEntity, or another Java type, these return types are mapped to a response entity body as follows:
void Results in an empty entity body with a 204 status code.
Response Results in an entity body mapped from the entity property of the Response with the status code specified by the status property of the Response. A null return value results in a 204 status code. If the status property of the Response is not set: a 200 status code is used for a non-null entity property and a 204 status code is used if the entity property is null.
GenericEntity Results in an entity body mapped from the Entity property of the GenericEntity. If the return value is not null a 200 status code is used, a null return value results in a 204 status code.
Other Results in an entity body mapped from the class of the returned instance. If the return value is not null a 200 status code is used, a null return value results in a 204 status code.
[...]
And since you are using an exception, the following section applies (emphasis mine):
3.3.4 Exceptions
A resource method, sub-resource method or sub-resource locator may throw any checked or unchecked exception. An implementation MUST catch all exceptions and process them as follows:
- Instances of WebApplicationException MUST be mapped to a response as follows. If the response property of the exception does not contain an entity and an exception mapping provider (see section 4.4) is available for WebApplicationException an implementation MUST use the provider to create a new Response instance, otherwise the response property is used directly. The resulting Response instance is then processed according to section 3.3.3.
[...]
So you should either return null, void or build a 204 response. You throw exceptions only if it's an exceptional case in your application and throwing the exception makes this clear.
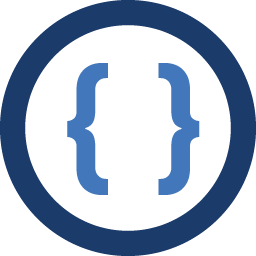
Admin
Updated on January 13, 2020Comments
-
Admin over 4 years
I am using jersey framework to develop RESTful web service. I am throwing various HTTP status codes with response using following code:
public class RestNoContentException extends WebApplicationException { public RestNoContentException(String message) { super(Response.status(Status.NO_CONTENT) .entity(message).type("text/plain") .build()); } }
While testing the REST web service using Firefox Mozilla rest client tool, it is displaying
200 OK
status instead of204 NO CONTENT
. I am handling the other status codes the same way I am doing for status code204
. Other status codes are appearing properly on rest client tool but when to show204
status code, it is showing200 OK
status code.Can someone please help me out here? what am I missing?