How to Undo and Redo in C# (Rich Text Box)
Solution 1
Ok, I'll post some code to help you get started. first you need to listen for the TextChanged
event.
textBox1.TextChanged += new EventHandler(textBox1_TextChanged);
and you need to declare a stack in the class
Stack<string> undoList = new Stack<string>();
In the text changed handler, you need to add the contents of the textbox to the stack
void textBox1_TextChanged(object sender, EventArgs e)
{
undoList.Push(textBox1.Text);
}
Then you need to handle the undo, so you could simply use CTRL-Z
textBox1.KeyDown += new KeyEventHandler(textBox1_KeyDown);
void textBox1_KeyDown(object sender, KeyEventArgs e)
{
if(e.KeyCode == Keys.Z && (e.Control)) {
textBox1.Text = undoList.Pop();
}
}
Solution 2
You can have the RichTextBox build the undo stack for you word by word, plus keep track of the caret position, by handling one of the KeyDown-, KeyPress- or KeyUp events like this:
private void rtb_KeyDown(object sender, KeyEventArgs e)
{
// Trick to build undo stack word by word
RichTextBox rtb = (RichTextBox)sender;
if (e.KeyCode == Keys.Space)
{
this.SuspendLayout();
rtb.Undo();
rtb.Redo();
this.ResumeLayout();
}
// eztnap
}
Since the RichTextBox does the job for you, you just have to call rtb.Undo()
or rtb.Redo()
from wherever you need to.
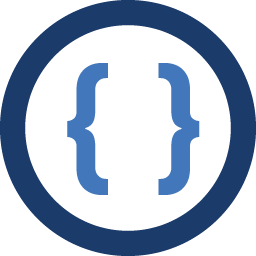
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
I've been trying to get undo and redo working in my text editor for about 3 days now. It's doing my head in.
I have a text box (named
richTextBoxPrintCtrl1
), which I would like to be able to undo and *redo *(word by word).So if I click the undo button, it undoes the last word. And if I then clicked the redo button, it redoes the last word.
Could somebody help me get this working?
richTextBoxPrintCtrl1.Undo();
doesn't work very well. It deletes everything typed in the text box.Thanks in advance for your help.
I know this question has been asked many times before, but I can't get it working using the information from the questions I've browsed here on SO.