How to update webpack config for a react project created using create-react-app?
Solution 1
No need to run npm run eject
Step 1
npm install react-app-rewired --save-dev
Step 2
Add config-overrides.js
to the project root directory.(NOT ./src)
// config-overrides.js
module.exports = function override(config, env) {
// New config, e.g. config.plugins.push...
return config
}
Step 3
'Flip' the existing calls to react-scripts in npm scripts for start, build and test
/* package.json */
"scripts": {
- "start": "react-scripts start",
+ "start": "react-app-rewired start",
- "build": "react-scripts build",
+ "build": "react-app-rewired build",
- "test": "react-scripts test",
+ "test": "react-app-rewired test",
"eject": "react-scripts eject"
}
Step 4
Restart your app. Done
Solution 2
Option 1 - Eject your CRA
If you've just created your app using CRA, and haven't made big changes to it, you could use npm run eject
- more about it here
Keep in mind that there is no going back (except by commits, of course) after doing this. This will basically provide you with webpack file and other files which are currently 'hidden' in CRA
Some critiques and second thoughts about this method here
Option 2 - React App Rewired
This might be the right choice for you. This allows you to extend your current webpack without ejecting, or messing up / making too many changes at your project as npm run eject
will. Take a look at the package here
A great tutorial by Egghead.io using react-app-rewired
here
Solution 3
I solved this problem by running a script between yarn install and yarn build. After yarn install the webpack.config.json file is generated, then immediately run a script on Node that modifies it, and then run the build.
My code:
custom.webpack.config.js
const fs = require('fs')
// WebPack.config File
const fileConfig = 'node_modules/react-scripts/config/webpack.config.js'
new Promise((resolve) => {
fs.readFile(fileConfig, 'utf8', function (err, data) {
if (err) {
return console.log(err)
}
resolve(data)
})
}).then((file) => {
let CodeAsString = "Code as String to save"
let regexCode = /YourCodeRegex}/g
let result = file.replace(regexCode, CodeAsString)
fs.writeFile(fileConfig, result, function (err) {
if (err) return console.log(err)
console.log('The webpack.config file was modifed!')
})
})
So, now do you need to edit the package.json to include this code in the process:
"scripts": {
"prestart": "node custom.webpack.config.js",
"prebuild": "node custom.webpack.config.js",
"start": "react-scripts start",
"build": "react-scripts build"
}
Done! :)
Solution 4
Webpack configuration is being handled by react-scripts. I assume that you don't want to eject and just want to look at the config, you will find them in /node_modules/react-scripts/config
webpack.config.dev.js. //used by `npm start`
webpack.config.prod.js //used by `npm run build`
Solution 5
https://www.npmjs.com/package/react-app-rewired
Complete answer is :
How to rewire your create-react-app
project
Create your app using create-react-app
and then rewire it.
- Install
react-app-rewired
Forcreate-react-app 2.x
with Webpack 4:
npm install react-app-rewired --save-dev
For create-react-app 1.x
or react-scripts-ts
with Webpack 3:
npm install [email protected] --save-dev
- Create a
config-overrides.js
file in the root directory
/* config-overrides.js */ module.exports = function override(config, env) { //do stuff with the webpack config... return config; }
like this:
+-- your-project
| +-- config-overrides.js
| +-- node_modules
| +-- package.json
| +-- public
| +-- README.md
| +-- src
for example :
module.exports = function override(config, env) { // New config, e.g. config.plugins.push... config.module.rules = [...config.module.rules, { test: /\.m?js/, resolve: { fullySpecified: false } } ] return config }
- 'Flip' the existing calls to react-scripts in npm scripts for start, build and test
from:
/* package.json */
"scripts": { "start": "react-scripts start", "build": "react-scripts build", "test": "react-scripts test", "eject": "react-scripts eject" }
To:
/* package.json */
"scripts": { "start": "react-app-rewired start", "build": "react-app-rewired build", "test": "react-app-rewired test", "eject": "react-scripts eject" }
Note: Do NOT flip the call for the eject script. That gets run only once for a project, after which you are given full control over the webpack configuration making react-app-rewired no longer required. There are no configuration options to rewire for the eject script.
- Start the Dev Server
npm start
- Build your app
npm run build
Related videos on Youtube
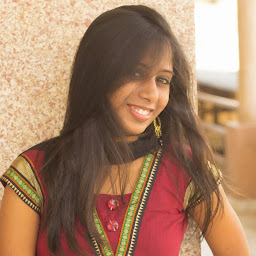
Deepshikha Mohanta
Updated on July 09, 2022Comments
-
Deepshikha Mohanta almost 2 years
I have created a react project using create-react-app. Now I need to update the webpack config, but I don't find the file anywhere. Do I need to create this file myself or what is the process? I am new to react and not really sure how to proceed from here.
-
strg over 3 yearsAn another option can be craco
-
BrS almost 3 yearsThis is not good. It will revert to original configuration after npm install.
-
Mohamed Jakkariya almost 3 yearsI was using it still now I'm not got any issues with that. I think you are not trying. If you're not okay with this go and install some other dependencies. That's fine :) Don't say anything without try.
-
Butri about 2 yearsdid not work for me
-
RiverTwilight about 2 years@Butri As the answer is simlified it's recommended for you to check the full doc. :-)