How to upload file in spring?
26,258
Solution 1
in your controller you need to specify that you are expecting mutlipart
using
consumes = {"multipart/form-data"}
and to ge the file name using getOriginalFileName()
@RequestMapping(method = RequestMethod.POST, consumes = {"multipart/form-data"})
public String importQuestion(@Valid @RequestParam("uploadedFileName")
MultipartFile multipart, BindingResult result, ModelMap model) {
logger.debug("Post method of uploaded Questions ");
logger.debug("Uploaded file Name : " + multipart.getOriginalFilename());
return "importQuestion";
}
Also in your html the name of your input of type file should be the same as the RequestParam "uploadedFileName"
<input type="file" name="uploadFileName" id="fileToUpload" required="" >
change it to
<input type="file" name="uploadedFileName" id="fileToUpload" required="" >
Solution 2
You can also use MutlipartFile in order to upload file as follow.
@RequestMapping(value = "/uploadFile", method = RequestMethod.POST)
@ResponseBody
public String uploadFile(@RequestParam("file") MultipartFile file) {
try {
String uploadDir = "/uploads/";
String realPath = request.getServletContext().getRealPath(uploadDir);
File transferFile = new File(realPath + "/" + file.getOriginalFilename());
file.transferTo(transferFile);
} catch (Exception e) {
e.printStackTrace();
return "Failure";
}
return "Success";
}
You don't need to use spring form for file upload, you can do it with plain HTML
<html>
<body>
<h2>Spring MVC file upload using Annotation configuration Metadata</h2>
Upload File :
<form name="fileUpload" method="POST" action="uploadFile" enctype="multipart/form-data">
<label>Select File</label> <br />
<input type="file" name="file" />
<input type="submit" name="submit" value="Upload" />
</form>
</body>
</html>
You need to configure MultipartResolver object in yours application configuration as follow
@Bean(name="multipartResolver")
public CommonsMultipartResolver multipartResolver() {
CommonsMultipartResolver multi = new CommonsMultipartResolver();
multi.setMaxUploadSize(100000);
return multi;
}
You can follow complete tutorial on how to upload file in Spring Framework Upload File in Spring MVC framework
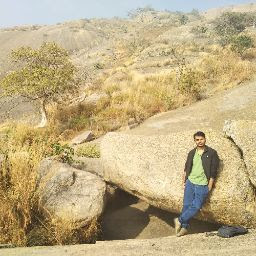
Author by
Anupam Choudhary
Updated on July 09, 2022Comments
-
Anupam Choudhary almost 2 years
I am not able to get the file name in spring controller
<form:form method="post" modelAttribute="sampleDetails" enctype="multipart/form-data"> <input type="file" name="uploadedFileName" id="fileToUpload" required="" > <input type="submit" name="import_file" value="Import File" id="" /> </form:form>
Its my post method in controller
@RequestMapping(method = RequestMethod.POST) public String importQuestion(@Valid @RequestParam("uploadedFileName") MultipartFile multipart, @ModelAttribute("sampleDetails") SampleDocumentPojo sampleDocument, BindingResult result, ModelMap model) { logger.debug("Post method of uploaded Questions "); logger.debug("Uploaded file Name : " + multipart.getName()); return "importQuestion"; }
After submit get the warning message.
warning [http-nio-8080-exec-9] WARN org.springframework.web.servlet.PageNotFound - Request method 'POST' not supported [http-nio-8080-exec-9] WARN org.springframework.web.servlet.mvc.support.DefaultHandlerExceptionResolver - Handler execution resulted in exception: Request method 'POST' not supported