How to use an overridden constant in an inheritanced class
Solution 1
class A
CONST = 'A'
def initialize
puts self.class::CONST
end
end
class B < A
CONST = 'B'
end
A.new # => 'A'
B.new # => 'B'
Solution 2
I had a few issues with the solution by Konstantin Haase. When accessing the constant in an instantiated object of the inheriting class, the parent constant was used.
I had to explicitly refer to the class.
self.class::CONST
cheers
Solution 3
Sorry I couldn't get the code formatting to work in a 'comment' only in an 'answer' but this is in response to akostadinov's question to Hendrik "how is this different from his [Konstantin's] answer?"
I'd guess Hendrik was trying to access the constant from methods in his inheriting class & that depends on if it's an instance or static method. It seems to behave as you'd expect in an instance method. But maybe or maybe not how you'd expect for a static method. Even if that's not what Hendrik meant, this may be worth noting:
If you have the exact class definitions as Konstantin, but you add a method to class A like this:
def self.print_const
puts CONST
end
Then you get A both times:
A.print_const # prints A
B.print_const # prints A
However if you define the method in A by referencing the class:
def self.print_const
puts self::CONST
end
Then you get:
A.print_const # prints A
B.print_const # prints B
Solution 4
In case anyone finds this and is using module extension instead, just use
self::CONST
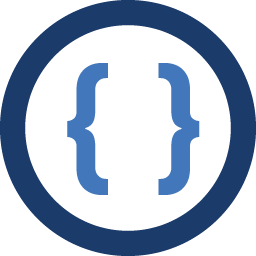
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
given this code:
class A CONST = 'A' def initialize puts CONST end end class B < A CONST = 'B' end A.new # => 'A' B.new # => 'A'
I'd like
B
to use theCONST = 'B'
definition, but I don't know how. Any ideas?Greetings
Tom
-
nohat over 13 yearsSo... this means there is no way to do it without changing everywhere in class A that uses CONST?
-
Konstantin Haase over 13 yearsYes. Constant lookup is usually bound at compile time.
-
akostadinov over 8 yearshow is this different from his answer?