how to use assertj extracting map property
Solution 1
AssertJ has entry() method. You can assert map value like this.
assertThat(list)
.extracting("myMap")
.contains(entry("foo1", "bar1"), entry("foo2", "bar2"));
Here's javadoc : http://joel-costigliola.github.io/assertj/core/api/org/assertj/core/data/MapEntry.html
Solution 2
The simplest way to assert the contents of your map is chaining the extracting
method:
MyObj o1 = new MyObj();
o1.getMyMap().put("foo", "Hello");
o1.getMyMap().put("bar", "Bye");
MyObj o2 = new MyObj();
o2.getMyMap().put("foo", "Hola");
o2.getMyMap().put("bar", "Adios");
List<MyObj> myObjs = Arrays.asList(o1, o2);
assertThat(myObjs).extracting("myMap").extracting("foo").contains("Hello", "Hola");
assertThat(myObjs).extracting("myMap").extracting("bar").contains("Bye", "Adios");
Solution 3
Extracting feature is documented here: http://joel-costigliola.github.io/assertj/assertj-core-features-highlight.html#extracted-properties-assertion
You have executable examples in assertj-examples, and specifically in IterableAssertionsExamples.
Hope it helps !
Solution 4
If you want to use the containsKey
method, you must know the type of the key at compile time (that is, you can't rely on generics). Supposing myMap
is a Map<String, Long>
you could do:
assertThat(list)
.extracting("myMap")
.asInstanceOf(InstanceOfAssertFactories.map(String.class, Long.class))
.containsKey("key");
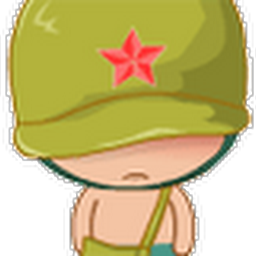
lhoak
Updated on June 04, 2022Comments
-
lhoak almost 2 years
I am using
AssertJ
. I have a class likeMyObj
. And I have aList
ofMyObj
.Class MyObj { ... Map<K,V> myMap; ... }
When I use:
assertThat(list).extracting("myMap")
, I cannot use.containsKey()
method.- I also tried using
assertThat(list).extracting("myMap", Map.class)
, but it does not work either.
What is the right way of using it?