How to use bootstrap css tables to display data from MySQL tables?
Solution 1
Try this
<?php
$hostname = '192.xx.xxx.xx';
$username = 'Mitchyl';
$password = 'root1323';
$dbname = 'test';
try {
$dbh = new PDO("mysql:host=$hostname;dbname=$dbname", $username, $password);
$sql = $dbh->prepare("SELECT * FROM increment");
if($sql->execute()) {
$sql->setFetchMode(PDO::FETCH_ASSOC);
}
}
catch(Exception $error) {
echo '<p>', $error->getMessage(), '</p>';
}
?>
<div id="content">
<table>
<?php while($row = $sql->fetch()) { ?>
<tr>
<td><?php echo $row['column1_name']; ?></td>
<td><?php echo $row['column2_name']; ?></td>
<td><?php echo $row['column3_name']; ?></td>
...etc...
</tr>
<?php } ?>
</table>
</div>
Solution 2
Let's say your sql returns an array named $data
and its format is sth like $data = [['name' => 'name1'], ['name' => 'name2'], ...];
.
//First declare your data array
$data = [];
// Then execute the query
$result = $mysqli->query($sql)
// Then read the results and create your $data array
while($row = $result->fetch_array())
{
$data[] = $row;
}
Now that you have your data check if it is empty or not and then use foreach to present the results.
<?php if(empty($data)): ?>
<h1>No results were found!</h1>
<?php else: ?>
<h1><?= count($data) ?> results were found!</h1>
<table class="table">
<thead>
<th>#</th>
<th>Name</th>
</thead>
<tbody>
<?php foreach ($data as $key => $value): ?>
<tr>
<td><?= ++$key ?></td>
<td><?= $value['name'] ?></td>
</tr>
<?php endforeach; ?>
</tbody>
</table>
<?php endif; ?>
Of course you can add any class you like to the table apart from the default one that bootstrap uses (.table).
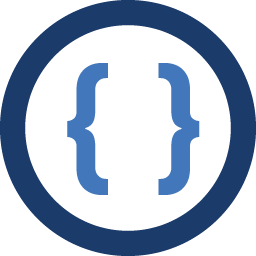
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm trying to make it so that when a button is clicked on my main page, a php script takes action and fetches the information from the SQL tables, and displays it in a HTML/CSS table.
Here's the code of my main page -
<form id="myForm" action="select.php" method="post"> <button type="submit" class="btn btn-info" > <span class="glyphicon glyphicon-tint"></span> View </button> <br /> <span class="badge alert-info"> Find out what is currently in the database. </span><br /> </form> <br /> <br />
And here's what I currently have in my select.php -
<?php /*** mysql hostname ***/ $hostname = '192.xx.xxx.xx'; /*** mysql username ***/ $username = 'Mitchyl'; /*** mysql password ***/ $password = 'root1323'; /*** database name ***/ $dbname = 'test'; try { $dbh = new PDO("mysql:host=$hostname;dbname=$dbname", $username, $password); /*** The SQL SELECT statement ***/ $sql = "SELECT * FROM increment"; } catch(PDOException $e) { echo $e->getMessage(); } ?>
I just don't know how to take the data from the SQL query and put it inside a HTML table.
Any suggestions would be great! Thanks!
-
Admin almost 10 yearsHi, I tried this, and used "<table class="table"> to make it look a bit better, and it looks like this - i.imgur.com/E5zsHjK.png Do you know of a way to format it?
-
Admin almost 10 yearsHow would I make sure the returns are labeled $data? Thanks for the reply though, I might be starting to grasp this concept haha.
-
Milan and Friends almost 10 yearsJust CSS e.g.
table { background: #eee; border: 1px solid #666; } td { font-size: 12px; color: #666; }
-
Admin almost 10 yearsI'm still having this weird issue, it always looks like junk. Using CSS and formatting doesn't change anything. Hmm.
-
Milan and Friends almost 10 yearsDo you use inline styling or calling external CSS document?
-
Admin almost 10 yearsAh, I'm mostly new to this so forgive me if I can't explain it very well. I'm using inline(I think). What I just found out is that it's making a new table for every entry in the table. I set the borders and everything, but I don't know how to make it so that it doesn't take up the entire page. That, and of course the fact that it's making a new table for every entry in the database...
-
Milan and Friends almost 10 yearsIt's not making a new table just table row, set width & height in CSS for table e.g.
table { width: 600px; height: 800px; }
to prevent table from taking whole space in document. -
Admin almost 10 yearsOh! Thanks so much, that fixed that issue. Now I have one last question. Before each new row in the table, it says the name of the table(eg."Card ID", "Card Hash"). Screenshot - i.imgur.com/BZy6g0c.png Any idea why this is or how to resolve it?
-
Milan and Friends almost 10 yearsStrange...I'm not sure what would cousing that.
-
Admin almost 10 yearsIt's alright. I don't fully know the rules to this website yet, would it be okay if I posted this as a new question?