How to use C++ to Copy A Directory
Solution 1
As of today, C++17's <filesystem>
is the proper solution.
#include <filesystem>
int main()
{
std::filesystem::copy("C:/Users/", "E:/Backup/");
}
The slashes (/
) are interpreted as directory separators, this is easier to read than a double back-slash (\\
).
Solution 2
1) include .h files:
#include <csystem>
2) write cmd:
system("copy c:\users\ e:\Backup\");
Tips: You can write everything in " " , just like you copy directorys in cmd.
Solution 3
The approach using System will not be platform independent. I strongly recommend using boost::filesystem for such tasks.
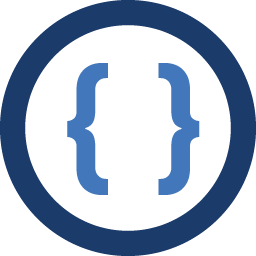
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I have made a backup program using c++, but it uses the System() command to batch copy files.
I am looking for a way to copy an entire directory (this does not need to create any directories, just copy them). Or alternatively, copy everything within a directory.
For example, I want to copy
C:\Users\ to E:\Backup\ Or C:\Users\* to E:\Backup\
.If possible could you include an example in your answer.
Thanks a lot!
-
KingsOfTechENG over 11 yearsfirst step is : # include <csystem>
-
harper over 11 yearsWhen you read the question you find that the OP has solved the task to call
system()
.