How To use ExecuteSqlCommandAsync
13,501
Your code is fine. It just needs to be inside an async
method:
public async Task FooAsync()
{
if (OldMarketRightsIDs.Count > 0)
{
TaskReturn = Data.MK3Model.Database.ExecuteSqlCommandAsync("DELETE FROM TitleMarketRights WHERE ID in (" + string.Join(", ", OldMarketRightsIDs) + ")");
}
var NewMarketRights = MarketRights.Select(m => new
{
Key = m.Key,
Value = m.Value.Except(CurrentMarketRights[m.Key].Select(c => c.FK_ProductRight).ToList())
}).ToList();
foreach (var mr in NewMarketRights)
{
foreach (var ProdID in mr.Value)
{
Data.MK3Model.TitleMarketRights.Add(new TitleMarketRight { FK_MarketID = (mr.Key == 0) ? null : (int?)mr.Key, FK_TitleID = ID, FK_ProductRight = ProdID });
}
}
var i = await TaskReturn;
}
That's what allows you to use await
. Behind the scenes a state machine is built that handles the asynchronous nature of you method. The method needs to have the async
keyword and return a Task
or Task<T>
if you have a return value.
If you would want to have it as a lambda expression then:
Func<Task> fooAsync = async () =>
{
if (OldMarketRightsIDs.Count > 0)
{
TaskReturn = Data.MK3Model.Database.ExecuteSqlCommandAsync("DELETE FROM TitleMarketRights WHERE ID in (" + string.Join(", ", OldMarketRightsIDs) + ")");
}
var NewMarketRights = MarketRights.Select(m => new
{
Key = m.Key,
Value = m.Value.Except(CurrentMarketRights[m.Key].Select(c => c.FK_ProductRight).ToList())
}).ToList();
foreach (var mr in NewMarketRights)
{
foreach (var ProdID in mr.Value)
{
Data.MK3Model.TitleMarketRights.Add(new TitleMarketRight { FK_MarketID = (mr.Key == 0) ? null : (int?)mr.Key, FK_TitleID = ID, FK_ProductRight = ProdID });
}
}
var i = await TaskReturn;
};
var task = fooAsync();
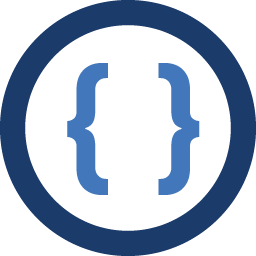
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I've been trying to figure out
ExecuteSqlCommandAsync
, which I haven't been able to find any good documentation on.if (OldMarketRightsIDs.Count > 0) { //This is where I want to have the task Begin TaskReturn = Data.MK3Model.Database.ExecuteSqlCommandAsync("DELETE FROM TitleMarketRights WHERE ID in (" + string.Join(", ", OldMarketRightsIDs) + ")"); } //This is the Code I want To execute in between var NewMarketRights = MarketRights.Select(m => new { Key = m.Key, Value = m.Value.Except(CurrentMarketRights[m.Key].Select(c => c.FK_ProductRight).ToList()) }).ToList(); foreach (var mr in NewMarketRights) { foreach (var ProdID in mr.Value) { Data.MK3Model.TitleMarketRights.Add(new TitleMarketRight { FK_MarketID = (mr.Key == 0) ? null : (int?)mr.Key, FK_TitleID = ID, FK_ProductRight = ProdID }); } } //This is where I want to wait until the task is complete before continuing var i = await TaskReturn;
This Example doesn't compile, it gets an error await operator can only be used with an async Method. Any Ideas on how to get this working