How to use Firebase invisible reCAPTCHA for angular web app?
Here is a working example with invisible reCAPTCHA:
https://github.com/firebase/quickstart-js/blob/master/auth/phone-invisible.html
In your code, after you call signInWithPhoneNumber
, invisible reCAPTCHA should either display a challenge which when solved, resolves the pending promise with the confirmationResult
, or directly resolves without showing any challenge.
When that promise resolves with confirmationResult
, you should ask the user for the SMS code.
You then call confirmationResult.confirm(code)
This will complete sign in of the user.
firebase
.auth()
.signInWithPhoneNumber(phoneNumber, appVerifier)
.then(function(confirmationResult) {
var code = window.prompt("Please enter your code");
return confirmationResult.confirm(code);
})
.then(function(result) {
// User is signed in now.
})
.catch(function(error) {
console.log("error", error);
});
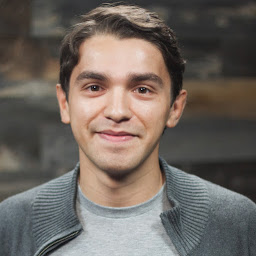
alphapilgrim
Focused on all things web with a keenness for front-end development -- currently, obsessed with performance and optimizations. A shared love for both code and aesthetics. Ionic / Angular/ AngularJS / Typescript / Firebase / Node / Java SOreadytohelp
Updated on June 09, 2022Comments
-
alphapilgrim about 2 years
Hoping to see a working example. Searched quite a bit, can't seem to get it working locally. Always shows privacy terms logo & user has to interact with captcha.
Here is a codepen I've created to speed things up.
From the Firebase-Docs:
Use invisible reCAPTCHA
To use an invisible reCAPTCHA, create a RecaptchaVerifier object with the size parameter set to invisible, specifying the ID of the button that submits your sign-in form.
window.recaptchaVerifier = new firebase.auth.RecaptchaVerifier('sign-in-button', { 'size': 'invisible', 'callback': function(response) { // reCAPTCHA solved, allow signInWithPhoneNumber. onSignInSubmit(); } });
Basically what I have in this views on init:
window.recaptchaVerifier = new firebase.auth.RecaptchaVerifier('phone-sign-in-recaptcha', { 'size': 'invisible', 'callback': function(response) { // reCAPTCHA solved - will proceed with submit function console.log(response); }, 'expired-callback': function() { // Reset reCAPTCHA? } });
<form name="mobile" novalidate> <label class="item item-input"> <i class="icon placeholder-icon"></i> <input type="tel" name="number" ng-model="$ctrl.user.mobile"> </label> <button id="sign-in-button" ng-click="$ctrl.onSignInSubmit(user.mobile)"></button> </form> <div id="phone-sign-in-recaptcha"></div>
This is the function called on submit:
ctrl.onSignInSubmit = function() { var phoneNumber = '+1' + ctrl.user.mobile; var appVerifier = window.recaptchaVerifier; firebase.auth() .signInWithPhoneNumber(phoneNumber, appVerifier) .then(function(confirmationResult) { ctrl.hasCodeToVerify = true; console.log('confirmationResult', confirmationResult); window.confirmationResult = confirmationResult; }).catch(function(error) { console.log('error', error); }); };
-
alphapilgrim over 6 yearsI had taken a look at that quick start, it has a render method I'm not sure if its suppose to actually render the challenge or autocomplete it. i tried using it in my code. Either not usuing it correctly or not going to solve the challenge. Ill make a codepen so maybe you/other can help through there.
-
alphapilgrim over 6 yearsI've seen your posts on other firebase and ionic questions. seems like this has something to do with originating URL. most of them get an HTTP error message, with the invisible captcha.
-
bojeil over 6 yearsHey @alphapilgrim, you don't seem to ask the user for the SMS code. I added a simple example showing how to do this.
-
alphapilgrim over 6 yearsquite right @bojeil! I had the confirmation in a different function with different click event. Thank you so much! I was about to remove this piece from the app.