How to use Java 8 `Files.find` method?
13,341
Solution 1
Path.getFilename()
does not return a String, but a Path object, do this:
getFilename().toString().equals("workspace")
Solution 2
Use the following and look at the console. Maybe none of your files contains workspace
in it
Files.find(p,maxDepth,(path, basicFileAttributes) -> {
if (String.valueOf(path).equals("workspace")) {
System.out.println("FOUND : " + path);
return true;
}
System.out.println("\tNOT VALID : " + path);
return false;
});
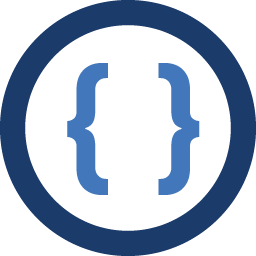
Author by
Admin
Updated on June 24, 2022Comments
-
Admin almost 2 years
I am trying to write an app to use
Files.find
method in it.Below program works perfectly :
package ehsan; /* I have removed imports for code brevity */ public class Main { public static void main(String[] args) throws IOException { Path p = Paths.get("/home/ehsan"); final int maxDepth = 10; Stream<Path> matches = Files.find(p,maxDepth,(path, basicFileAttributes) -> String.valueOf(path).endsWith(".txt")); matches.map(path -> path.getFileName()).forEach(System.out::println); } }
This works fine and gives me a list of files ending with
.txt
( aka text files ) :hello.txt ...
But below program does not show anything :
package ehsan; public class Main { public static void main(String[] args) throws IOException { Path p = Paths.get("/home/ehsan"); final int maxDepth = 10; Stream<Path> matches = Files.find(p,maxDepth,(path, basicFileAttributes) -> path.getFileName().equals("workspace")); matches.map(path -> path.getFileName()).forEach(System.out::println); } }
But it does not show anything :(
Here is my home folder hiearchy (
ls
result) :blog Projects Desktop Public Documents Templates Downloads The.Purge.Election.Year.2016.HC.1080p.HDrip.ShAaNiG.mkv IdeaProjects The.Purge.Election.Year.2016.HC.1080p.HDrip.ShAaNiG.mkv.aria2 Music Videos Pictures workspace
So whats going wrong with
path.getFileName().equals("workspace")
? -
Admin almost 8 yearsThanks, Isn't it funny that getFileName() does not actually return file name ! ?
-
Admin almost 8 yearsAs shown in my Home directory hiearchy, I HAVE a
workspace
folder :) -
Admin almost 8 yearsThanks, It was very useful and user friendly :)
-
Yassin Hajaj almost 8 years@ehsan Youre welcome, I do not know if it is sarcasm but I wasn't being non friendly at all don't worry :) Hope it helped