How to use pagination on HTML tables?
Solution 1
It is a very simple and effective utility build in jquery to implement pagination on html table http://tablesorter.com/docs/example-pager.html
Download the plugin from http://tablesorter.com/addons/pager/jquery.tablesorter.pager.js
After adding this plugin add following code in head script
$(document).ready(function() {
$("table")
.tablesorter({widthFixed: true, widgets: ['zebra']})
.tablesorterPager({container: $("#pager")});
});
Solution 2
Many times we might want to perform Table pagination using jquery.Here i ll give you the answer and reference link
Jquery
$(document).ready(function(){
$('#data').after('<div id="nav"></div>');
var rowsShown = 4;
var rowsTotal = $('#data tbody tr').length;
var numPages = rowsTotal/rowsShown;
for(i = 0;i < numPages;i++) {
var pageNum = i + 1;
$('#nav').append('<a href="#" rel="'+i+'">'+pageNum+'</a> ');
}
$('#data tbody tr').hide();
$('#data tbody tr').slice(0, rowsShown).show();
$('#nav a:first').addClass('active');
$('#nav a').bind('click', function(){
$('#nav a').removeClass('active');
$(this).addClass('active');
var currPage = $(this).attr('rel');
var startItem = currPage * rowsShown;
var endItem = startItem + rowsShown;
$('#data tbody tr').css('opacity','0.0').hide().slice(startItem, endItem).
css('display','table-row').animate({opacity:1}, 300);
});
});
JSfiddle: https://jsfiddle.net/u9d1ewsh/
Solution 3
For me, best and simplest way, Bootply http://www.bootply.com/lxa0FF9yhw#
First include Bootstrap to your project
Then include javascript file in which you write this code:
$.fn.pageMe = function(opts){
var $this = this,
defaults = {
perPage: 7,
showPrevNext: false,
hidePageNumbers: false
},
settings = $.extend(defaults, opts);
var listElement = $this;
var perPage = settings.perPage;
var children = listElement.children();
var pager = $('.pager');
if (typeof settings.childSelector!="undefined") {
children = listElement.find(settings.childSelector);
}
if (typeof settings.pagerSelector!="undefined") {
pager = $(settings.pagerSelector);
}
var numItems = children.size();
var numPages = Math.ceil(numItems/perPage);
pager.data("curr",0);
if (settings.showPrevNext){
$('<li><a href="#" class="prev_link">«</a></li>').appendTo(pager);
}
var curr = 0;
while(numPages > curr && (settings.hidePageNumbers==false)){
$('<li><a href="#" class="page_link">'+(curr+1)+'</a></li>').appendTo(pager);
curr++;
}
if (settings.showPrevNext){
$('<li><a href="#" class="next_link">»</a></li>').appendTo(pager);
}
pager.find('.page_link:first').addClass('active');
pager.find('.prev_link').hide();
if (numPages<=1) {
pager.find('.next_link').hide();
}
pager.children().eq(1).addClass("active");
children.hide();
children.slice(0, perPage).show();
pager.find('li .page_link').click(function(){
var clickedPage = $(this).html().valueOf()-1;
goTo(clickedPage,perPage);
return false;
});
pager.find('li .prev_link').click(function(){
previous();
return false;
});
pager.find('li .next_link').click(function(){
next();
return false;
});
function previous(){
var goToPage = parseInt(pager.data("curr")) - 1;
goTo(goToPage);
}
function next(){
goToPage = parseInt(pager.data("curr")) + 1;
goTo(goToPage);
}
function goTo(page){
var startAt = page * perPage,
endOn = startAt + perPage;
children.css('display','none').slice(startAt, endOn).show();
if (page>=1) {
pager.find('.prev_link').show();
}
else {
pager.find('.prev_link').hide();
}
if (page<(numPages-1)) {
pager.find('.next_link').show();
}
else {
pager.find('.next_link').hide();
}
pager.data("curr",page);
pager.children().removeClass("active");
pager.children().eq(page+1).addClass("active");
}
};
You need to give an id to the tbody of your table and to add a 'div' after the table for the pagination
<table class="table" id="myTable">
<thead>
<tr>
<th>...</th>
</tr>
</thead>
<tbody id="myTableBody">
</tbody>
</table>
<div class="col-md-12 text-center">
<ul class="pagination pagination-lg pager" id="myPager"></ul>
</div>
When your table's data is loaded, just call this
$('#myTableBody').pageMe({pagerSelector:'#myPager',showPrevNext:true,hidePageNumbers:false,perPage:4});
where the 'perPage' value is to set how many elements per page you want to have.
Solution 4
Pure js. Can apply it to multiple tables at once. Aborts if only one page is required. I used anushree as my starting point.
Sorry to the asker, obviously this is not a simplePagignation.js solution. However, it's the top google result when you type "javascript table paging", and it's a reasonable solution to many who may be considering a library but unsure whether to go that route or not.
Use like this:
addPagerToTables('#someTable', 8);
Requires no css, though it may be wise to initially hide table tBody rows in css anyway to prevent the effect of rows showing then quicky being hidden (not happening with me right now, but it's something I've seen before).
The code:
function addPagerToTables(tables, rowsPerPage = 10) {
tables =
typeof tables == "string"
? document.querySelectorAll(tables)
: tables;
for (let table of tables)
addPagerToTable(table, rowsPerPage);
}
function addPagerToTable(table, rowsPerPage = 10) {
let tBodyRows = table.querySelectorAll('tBody tr');
let numPages = Math.ceil(tBodyRows.length/rowsPerPage);
let colCount =
[].slice.call(
table.querySelector('tr').cells
)
.reduce((a,b) => a + parseInt(b.colSpan), 0);
table
.createTFoot()
.insertRow()
.innerHTML = `<td colspan=${colCount}><div class="nav"></div></td>`;
if(numPages == 1)
return;
for(i = 0;i < numPages;i++) {
let pageNum = i + 1;
table.querySelector('.nav')
.insertAdjacentHTML(
'beforeend',
`<a href="#" rel="${i}">${pageNum}</a> `
);
}
changeToPage(table, 1, rowsPerPage);
for (let navA of table.querySelectorAll('.nav a'))
navA.addEventListener(
'click',
e => changeToPage(
table,
parseInt(e.target.innerHTML),
rowsPerPage
)
);
}
function changeToPage(table, page, rowsPerPage) {
let startItem = (page - 1) * rowsPerPage;
let endItem = startItem + rowsPerPage;
let navAs = table.querySelectorAll('.nav a');
let tBodyRows = table.querySelectorAll('tBody tr');
for (let nix = 0; nix < navAs.length; nix++) {
if (nix == page - 1)
navAs[nix].classList.add('active');
else
navAs[nix].classList.remove('active');
for (let trix = 0; trix < tBodyRows.length; trix++)
tBodyRows[trix].style.display =
(trix >= startItem && trix < endItem)
? 'table-row'
: 'none';
}
}
Solution 5
you can use this function . Its taken from https://convertintowordpress.com/simple-jquery-table-pagination-code/
function pagination(){
var req_num_row=10;
var $tr=jQuery('tbody tr');
var total_num_row=$tr.length;
var num_pages=0;
if(total_num_row % req_num_row ==0){
num_pages=total_num_row / req_num_row;
}
if(total_num_row % req_num_row >=1){
num_pages=total_num_row / req_num_row;
num_pages++;
num_pages=Math.floor(num_pages++);
}
for(var i=1; i<=num_pages; i++){
jQuery('#pagination').append("<a href='#' class='btn'>"+i+"</a>");
}
$tr.each(function(i){
jQuery(this).hide();
if(i+1 <= req_num_row){
$tr.eq(i).show();
}
});
jQuery('#pagination a').click(function(e){
e.preventDefault();
$tr.hide();
var page=jQuery(this).text();
var temp=page-1;
var start=temp*req_num_row;
//alert(start);
for(var i=0; i< req_num_row; i++){
$tr.eq(start+i).show();
}
});
}
Related videos on Youtube
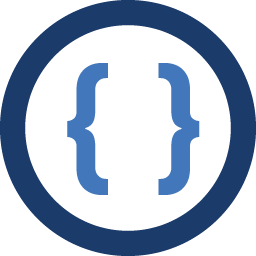
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am trying to use this Pagination library in my HTML table page (specifically light theme) but somehow I am not able to understand how to plugin this library in such a way in my HTML page so that I can have pagination code working in my HTML table..
Right now, I have HTML table without any pagination code working so my below HTML code will show you a table with all the rows in one page which is not what I want...
I want to show 6 items in my table in one page by using above pagination javascript.. And as soon as I click on second pagination tab, it should show me next six items and keep on going until it is finished..
Below is my full HTML code in which I have tried using the same above pagination javascript but it doesn't work for me..
<html> <head> <script src="http://code.jquery.com/jquery-1.9.1.min.js"></script> <script src="../jquery.simplePagination.js"></script> <link href="../simplePagination.css" rel="stylesheet" type="text/css" /> <style type="text/css"> table { width: 40em; margin: 2em auto; } thead { background: #000; color: #fff; } td { width: 10em; padding: 0.3em; } tbody { background: #ccc; } </style> <script> function test(pageNumber) { var page="#page-id-"+pageNumber; $('.select').hide() $(page).show() } </script> </head> <body bgcolor="#F8F8F8"> <table class="paginated"> <thead> <tr> <th>A</th> <th>B</th> <th>C</th> <th>D</th> </tr> </thead> <tbody> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> <tr> <td>1</td> <td>2</td> <td>3</td> <td>4</td> </tr> </tbody> </table> <div id="choose"> </div> <script language="javascript"> $(function() { $('#choose').pagination({ items: 20, itemsOnPage: 2, cssStyle: 'light-theme', onPageClick: function(pageNumber){test(pageNumber)} }); }); </script> </body> </html>
Any simple jsfiddle example basis on my above HTML code and also combined with above pagination javascript will help me understand better in how to imlement this js on the HTML tables..
Thanks for the help..
NOTE:-
I am only interested in simplePagination.js solution only which I am currently trying to implement
-
Admin over 10 yearsThanks Ashwin.. But I am specifically interested in that pagination library javascript solution.. Thanks for the help anyways...
-
Arun_SE almost 9 yearsya nice. if anyone wants without a plugin
-
Waqas Khan about 8 yearsUncaught TypeError: $(...).tablesorter is not a function gives me this error. i also include plugin file but still got this error
-
Jaylen over 7 yearsI am trying to this code. but every-time I run it I get this error in the console
TypeError: listElement.children is not a function
I tried to end my code with(jQuery);
since I put the code in a separate file but still getting the same error. -
jon over 7 yearsdid you include it in your html?
-
Robert over 7 yearsThank you! Saved me a whole lot of time.
-
ASalameh almost 7 yearsUse jQuery (1.2.1 or higher)
-
Akshay almost 6 yearsThanks for this.. one issue I am facing is when it paginates the scroll position resets to top.. any solution for that ?
-
bjornredemption almost 5 yearsadd an e.preventDefault(); right after the 'click' event to stop scrolling upwards
-
Above The Law over 4 yearsThat website is down.
-
Aaron John Sabu about 4 yearsSeems like the website does not exist any more
-
Christopher Smit about 4 yearsBrilliant! Had to modify it a little as I needed my pages to change based on clicking next or previous, but this was an excellent starting point!
-
Michael David over 2 yearsFor anybody wondering how to stop the scroll position from resetting to the top, change the href="#" in this line $('#nav').append('<a href="#" rel="'+i+'">'+pageNum+'</a> '); to href="javascript:void()". It prevents it from performing any default action.