How to use Refresh Token Google API in Python?
10,902
To refresh an Access Token, you call the Google OAuth endpoint passing in three parameters:
- Client ID
- Client Secret
- Refresh Token
This can be done very simply with a simple HTTP POST request.
Here is an example using curl:
set REFRESH_TOKEN=REPLACE_WITH_REFRESH_TOKEN
curl ^
--data client_id=%CLIENT_ID% ^
--data client_secret=%CLIENT_SECRET% ^
--data grant_type=refresh_token ^
--data refresh_token=%REFRESH_TOKEN% ^
https://www.googleapis.com/oauth2/v4/token
In Python using the requests library:
// Call refreshToken which creates a new Access Token
access_token = refreshToken(client_id, client_secret, refresh_token)
// Pass the new Access Token to Credentials() to create new credentials
credentials = google.oauth2.credentials.Credentials(access_token)
// This function creates a new Access Token using the Refresh Token
// and also refreshes the ID Token (see comment below).
def refreshToken(client_id, client_secret, refresh_token):
params = {
"grant_type": "refresh_token",
"client_id": client_id,
"client_secret": client_secret,
"refresh_token": refresh_token
}
authorization_url = "https://www.googleapis.com/oauth2/v4/token"
r = requests.post(authorization_url, data=params)
if r.ok:
return r.json()['access_token']
else:
return None
Note: This code will also return a refreshed ID Token if originally requested during the authorization request.
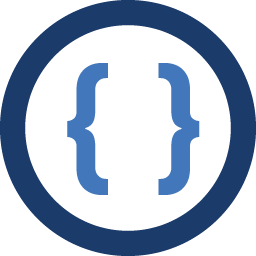
Author by
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
This function is to get authenticated service from Google. After
API_SERVICE_NAME = 'youtubereporting' API_VERSION = 'v1' CLIENT_SECRETS_FILE = "client_secret_929791903032-hpdm8djidqd8o5nqg2gk66efau34ea6q.apps.googleusercontent.com.json" SCOPES = ['https://www.googleapis.com/auth/yt-analytics-monetary.readonly'] def get_authenticated_service(): flow = InstalledAppFlow.from_client_secrets_file(CLIENT_SECRETS_FILE, SCOPES) credentials = flow.run_local_server() return build(API_SERVICE_NAME, API_VERSION, credentials=credentials)
But I want to use Refresh Token to automatically authenticate without opening a browser. Therefore add some codes into the function above to save Refresh Token:
def get_authenticated_service(): flow = InstalledAppFlow.from_client_secrets_file(CLIENT_SECRETS_FILE, SCOPES) credentials = flow.run_local_server() with open('refresh.token', 'w+') as f: f.write(credentials._refresh_token) print('Refresh Token:', credentials._refresh_token) print('Saved Refresh Token to file: refresh.token') return build(API_SERVICE_NAME, API_VERSION, credentials=credentials)
Okay, so after having the Refresh Token, How to use it? I tried to replace the refresh token
="ABCDEF456"
but it does not workdef get_authenticated_service(): return build(API_SERVICE_NAME, API_VERSION, credentials="ABCDEF456")
-
Admin over 5 yearsHow to import
requests
? -
Admin over 5 yearsOh I imported! but the code returned nothing
-
Ulysse Mizrahi almost 4 yearsthis does not work and fails with The request is missing a valid API key
-
John Hanley almost 4 years@UlysseMizrahi - API Keys and OAuth Tokens are different types of authorization. Create a new question showing how you created the tokens and the exact error message.
-
Satya about 3 years@Analytics POPSWW were you able to resolve your issue of not opening up the browser to authorize access to the GDrive files ? As I am stuck on a similar issue . Would be great if you could please share the steps to resolve it. I am having a oAuth2 client_secret.json which I am using to authorize and list files in the drive, I am then using the credentials.json file from service account to read the content. However, I would like to automate it without the need to authorize manually, and run the script from AWS EC2 instance.