How to use Regex.Replace to Replace Two Strings at Once?
15,919
Solution 1
Try
return Regex.Replace(filename, "[#']", "_");
Mind you, I'm not sure that a regex is likely to be faster than the somewhat simpler:
return filename.Replace('#', '_')
.Replace('\'', '_');
Solution 2
And just for fun, you can accomplish the same thing with LINQ:
var result = from c in fileName
select (c == '\'' || c == '#') ? '_' : c;
return new string(result.ToArray());
Or, compressed to a sexy one-liner:
return new string(fileName.Select(c => c == '\'' || c == '#' ? '_' : c).ToArray())
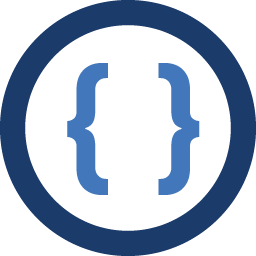
Author by
Admin
Updated on August 24, 2022Comments
-
Admin over 1 year
I have the following method that is replacing a "pound" sign from the file name but I want also to be able to replace the "single apostrophe ' " at the same time. How can I do it? This is the value of filename =Provider license_A'R_Ab#acus Settlements_1-11-09.xls
static string removeBadCharPound(string filename) { // Replace invalid characters with "_" char. //I want something like this but is NOT working //return Regex.Replace(filename, "# ' ", "_"); return Regex.Replace(filename, "#", "_"); }
-
Blindy almost 15 yearsIt has to be faster since you compile to regex's in the second example and parse the string (at least) twice (not to mention an extra string instance and the overhead of copying it over)
-
Patrick McDonald almost 15 yearsTested on my machine, for 100,000 iterations, RegEx took 677 ms, Replace took 143 ms.
-
Jon Skeet almost 15 years@Blindy: What makes you think the second example uses regular expressions at all?
-
Jon Skeet almost 15 years(Another alternative is to use a regular expression compiled once and stored in a static variable. I'd still generally prefer the String.Replace version for simplicity though.)
-
patjbs almost 15 yearsI've also found that if you can do it without a regular expression easily enough, it's invariably better to do so.
-
Pradeep Kumar over 11 years+1 for the String vs. Regex test. Regular Expressions are slow and should be used only for complex string matching. I wasn't surprised by your test results, and was expecting the same.