How to use Spring Boot Rest Data to return XML
Solution 1
RequestMapping annotation doesn't work on the repositoryies. Repository methods don't allow you to change the result format (default is JSON). If you want your service to return data in XML format then you need to create simple @Controller.
@Controller
public class RestEndpoint {
@Autowired
private SomeRepository someRepository;
@RequestMapping(value="/findByID", method=RequestMethod.GET, produces=MediaType.APPLICATION_XML_VALUE)
public @ResponseBody MyXmlAnnotatedObject findById(@Param("id") BigInteger id) {
return someRepository.findById(id);
}
}
UPD: Here is a link to the official Spring documentation: http://docs.spring.io/spring-data/rest/docs/2.1.4.RELEASE/reference/html/repository-resources.html
**3.6 The query method resource**
The query method resource executes the query exposed through an individual query method on the repository interface.
**3.6.1 Supported HTTP methods**
As the search resource is a read-only resource it supports GET only.
**GET**
Returns the result of the query execution.
**Parameters**
If the query method has pagination capabilities (indicated in the URI template pointing to the resource) the resource takes the following parameters:
page - the page number to access (0 indexed, defaults to 0).
size - the page size requested (defaults to 20).
sort - a collection of sort directives in the format ($propertyname,)+[asc|desc]?.
**Supported media types**
application/hal+json
application/json
Solution 2
Please don't blame me for necrophilia, but I have created an example that does exactly what you need: https://github.com/sergpank/spring-boot-xml
In brief you should tell to the platform that you need XML in the header of POST request (if you use a tool like Postman for testing):
Accept : application/xml
Content-Type : application/xml
OR it will be done automatically if you will set up XML message converter to RestTemplate:
public class RestfulClient {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate(Arrays.asList(new Jaxb2RootElementHttpMessageConverter()));
Contact random = restTemplate.getForObject("http://localhost:8080/contact/random", Contact.class);
System.out.println("Received: " + random);
Contact edited = restTemplate.postForObject("http://localhost:8080/contact/edit", random, Contact.class);
System.out.println("Edited: " + edited);
}
}
And don't forget to annotate you class with @XmlRootElement and @XmlElement annotations (if you prefer JAXB):
@AllArgsConstructor
@NoArgsConstructor
@ToString
@Setter
@XmlRootElement
public class Contact implements Serializable {
@XmlElement
private Long id;
@XmlElement
private int version;
@Getter private String firstName;
@XmlElement
private String lastName;
@XmlElement
private Date birthDate;
public static Contact randomContact() {
Random random = new Random();
return new Contact(random.nextLong(), random.nextInt(), "name-" + random.nextLong(), "surname-" + random.nextLong(), new Date());
}
}
Methods in Your controller should also have @RequestBody annotation to unmarshal XML and @ResponseBody annotation to marshal response back XML.
@Controller
@RequestMapping(value="/contact")
public class ContactController {
final Logger logger = LoggerFactory.getLogger(ContactController.class);
@RequestMapping("/random")
@ResponseBody
public Contact randomContact() {
return Contact.randomContact();
}
@RequestMapping(value = "/edit", method = RequestMethod.POST)
@ResponseBody
public Contact editContact(@RequestBody Contact contact) {
logger.info("Received contact: {}", contact);
contact.setFirstName(contact.getFirstName() + "-EDITED");
return contact;
}
}
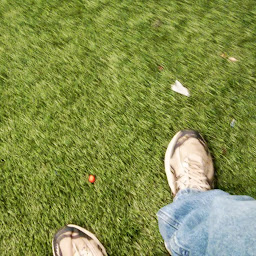
KRico
Updated on July 09, 2022Comments
-
KRico almost 2 years
I need the output of Spring Boot, Spring Data REST is XML,not JSON. I put in Repository:
@RequestMapping(value="/findByID", method=RequestMethod.GET, headers = { "Accept=application/xml" }, produces="application/xml") MyXmlAnnotatedObject findById(@Param("id") BigInteger id);
I also added the following to my pom dependancies
<dependency> <groupId>com.fasterxml.jackson.dataformat</groupId> <artifactId>jackson-dataformat-xml</artifactId> <version>2.4.3</version> </dependency> <dependency> <groupId>org.codehaus.woodstox</groupId> <artifactId>woodstox-core-asl</artifactId> <version>4.4.1</version> </dependency>
But when I try
http://localhost:9000/factset/search/findByID?id=18451
I still get JSON. I really need XML for my users Any Ideas?
-
Saurabh Bhoomkar almost 7 yearsGetting this error -> There was an unexpected error (type=Not Acceptable, status=406). Could not find acceptable representation
-
David Avendasora about 6 yearsThis answer does not apply to using Spring Data REST, which as of 3.0.6 specifically states "Currently, only JSON representations are supported." (docs.spring.io/spring-data/rest/docs/current/reference/html/…).