How to use watir-webdriver to wait for page load
Solution 1
I don't know if they're the best way, but this is how I'm handling this for waiting for an updating div
to clear:
while browser.div(:id=>"updating_div").visible? do sleep 1 end
This is how I handle waiting for something to display:
until browser.div(:id=>"some_div").exists? do sleep 1 end
Solution 2
Today's release adds an optional require that brings in some helpers for waiting for elements. These are not (at the moment) available in Watir 1.6, so be aware if you use both libraries side by side.
Check "AJAX and waiting for elements" in the Watir-webdriver Wiki for more information.
Solution 3
The best summary is found in "Waiting".
This is it in a nutshell:
require 'watir-webdriver'
b = Watir::Browser.start 'bit.ly/watir-webdriver-demo'
b.select_list(:id => 'entry_1').wait_until_present
b.text_field(:id => 'entry_0').when_present.set 'your name'
b.button(:value => 'Submit').click
b.button(:value => 'Submit').wait_while_present
Watir::Wait.until { b.text.include? 'Thank you' }
Solution 4
browser.wait_until
can be used.
It's more helpful because you can define what to wait for in the parameters (()
), as in:
browser.wait_until(browser.text.include("some text"))
Solution 5
This is how I wait for AJAX in my project:
ajax_loader = $b.element(:xpath => "//*[@id='spinner-modal-transparent' and @aria-hidden='true']/div/div/div/div/img[@alt='Ajax transparent loader']")
if ajax_loader.exists?
ajax_loader.wait_while_present(timeout=350)
else
puts "The AJAX loader was not present."
end
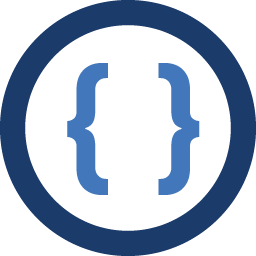
Admin
Updated on July 19, 2022Comments
-
Admin almost 2 years
Using watir-webdriver, how do I wait for a page to load after I click a link?
At the moment I am using:
sleep n
But this is not ideal as the page response varies so much.
Is there a way to test whether the page is ready or whether there is a certain element in the page? I understand in the normal Watir gem there is
Watir::Waiter.wait_until
or something similar, but I don't see this in the webdriver version.