How to validate multiple emails using Regex?
Solution 1
var email = "[A-Za-z0-9\._%-]+@[A-Za-z0-9\.-]+\.[A-Za-z]{2,4}";
var re = new RegExp('^'+email+'(;\\n*'+email+')*;?$');
[ "[email protected];[email protected]",
"[email protected];[email protected];",
"[email protected];\[email protected];\[email protected]",
"[email protected] [email protected]",
"[email protected],",
"[email protected]\[email protected]" ].map(function(str){
return re.test(str);
}); // [true, true, true, false, false, false]
Solution 2
This is how I'm doing it (ASP.Net app, no jQuery). The list of email addresses is entered in a multi-line text box:
function ValidateRecipientEmailList(source, args)
{
var rlTextBox = $get('<%= RecipientList.ClientID %>');
var recipientlist = rlTextBox.value;
var valid = 0;
var invalid = 0;
// Break the recipient list up into lines. For consistency with CLR regular i/o, we'll accept any sequence of CR and LF characters as an end-of-line marker.
// Then we iterate over the resulting array of lines
var lines = recipientlist.split( /[\r\n]+/ ) ;
for ( i = 0 ; i < lines.length ; ++i )
{
var line = lines[i] ; // pull the line from the array
// Split each line on a sequence of 1 or more whitespace, colon, semicolon or comma characters.
// Then, we iterate over the resulting array of email addresses
var recipients = line.split( /[:,; \t\v\f\r\n]+/ ) ;
for ( j = 0 ; j < recipients.length ; ++j )
{
var recipient = recipients[j] ;
if ( recipient != "" )
{
if ( recipient.match( /^([A-Za-z0-9_-]+\.)*[A-Za-z0-9_-]+\@([A-Za-z0-9_-]+\.)+[A-Za-z]{2,4}$/ ) )
{
++valid ;
}
else
{
++invalid ;
}
}
}
}
args.IsValid = ( valid > 0 && invalid == 0 ? true : false ) ;
return ;
}
Solution 3
There is no reason not to use split - in the same way the backend will obviously do.
return str.split(/;\s*/).every(function(email) {
return /.../.test(email);
}
For good or not-so-good email regular expressions have a look at Validate email address in JavaScript?.
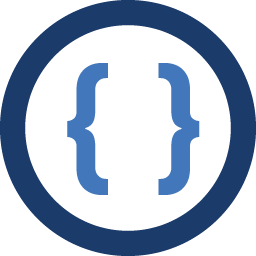
Admin
Updated on June 04, 2022Comments
-
Admin about 2 years
After a quick research on the Stackoverflow, I wasn't able to find any solution for the multiple email validation using regex (split JS function is not applicable, but some reason back-end of the application waits for a string with emails separated by
;
).Here are the requirements:
- Emails should be validated using the following rule:
[A-Za-z0-9\._%-]+@[A-Za-z0-9\.-]+\.[A-Za-z]{2,4}
- Regex should accept
;
sign as a separator - Emails can be written on multiple lines, finishing with
;
- Regex may accept the end of the line as
;
I come up with this solution:
^[A-Za-z0-9\._%-]+@[A-Za-z0-9\.-]+\.[A-Za-z]{2,4}(?:[;][A-Za-z0-9\._%-]+@[A-Za-z0-9\.-]+\.[A-Za-z]{2,4}?)*
but it doesn't work for point #3-4
So here are cases that are OK:
1. [email protected];[email protected] 2. [email protected];[email protected]; 3. [email protected]; [email protected]; [email protected];
Here are cases that are definetely NOT OK:
1. [email protected] [email protected] 2. [email protected], 3. [email protected] [email protected]
All sort of help will be appreciated
- Emails should be validated using the following rule: