How to view an HTML file in the browser with Visual Studio Code
Solution 1
For Windows - Open your Default Browser - Tested on VS Code v 1.1.0
Answer to both opening a specific file (name is hard-coded) OR opening ANY other file.
Steps:
Use ctrl + shift + p (or F1) to open the Command Palette.
-
Type in
Tasks: Configure Task
or on older versionsConfigure Task Runner
. Selecting it will open the tasks.json file. Delete the script displayed and replace it by the following:{ "version": "0.1.0", "command": "explorer", "windows": { "command": "explorer.exe" }, "args": ["test.html"] }
Remember to change the "args" section of the tasks.json file to the name of your file. This will always open that specific file when you hit F5.
You may also set the this to open whichever file you have open at the time by using
["${file}"]
as the value for "args". Note that the$
goes outside the{...}
, so["{$file}"]
is incorrect. Save the file.
Switch back to your html file (in this example it's "text.html"), and press ctrl + shift + b to view your page in your Web Browser.
Solution 2
VS Code has a Live Server Extension that supports one click launch from status bar.
Some of the features:
- One Click Launch from Status Bar
- Live Reload
- Support for Chrome Debugging Attachment
Solution 3
@InvisibleDev - to get this working on a mac trying using this:
{
"version": "0.1.0",
"command": "Chrome",
"osx": {
"command": "/Applications/Google Chrome.app/Contents/MacOS/Google Chrome"
},
"args": [
"${file}"
]
}
If you have chrome already open, it will launch your html file in a new tab.
Solution 4
-
Open Extensions Sidebar (Ctrl + Shift + X)
-
Search for open in browser and install it
-
Right click on your html file, and select "Open in Browser" (Alt + B)
Solution 5
If you would like to have live reload you can use gulp-webserver, which will watch for your file changes and reload page, this way you don't have to press F5 every time on your page:
Here is how to do it:
-
Open command prompt (cmd) and type
npm install --save-dev gulp-webserver
Enter Ctrl+Shift+P in VS Code and type Configure Task Runner. Select it and press enter. It will open tasks.json file for you. Remove everything from it end enter just following code
tasks.json
{
"version": "0.1.0",
"command": "gulp",
"isShellCommand": true,
"args": [
"--no-color"
],
"tasks": [
{
"taskName": "webserver",
"isBuildCommand": true,
"showOutput": "always"
}
]
}
- In the root directory of your project add gulpfile.js and enter following code:
gulpfile.js
var gulp = require('gulp'),
webserver = require('gulp-webserver');
gulp.task('webserver', function () {
gulp.src('app')
.pipe(webserver({
livereload: true,
open: true
}));
});
- Now in VS Code enter Ctrl+Shift+P and type "Run Task" when you enter it you will see your task "webserver" selected and press enter.
Your webserver now will open your page in your default browser. Now any changes that you will do to your HTML or CSS pages will be automatically reloaded.
Here is an information on how to configure 'gulp-webserver' for instance port, and what page to load, ...
You can also run your task just by entering Ctrl+P and type task webserver
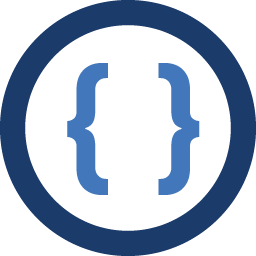
Admin
Updated on January 05, 2022Comments
-
Admin over 2 years
How can I view my HTML code in a browser with the new Microsoft Visual Studio Code?
With Notepad++ you have the option to Run in a browser. How can I do the same thing with Visual Studio Code?
-
Admin about 9 yearsI got this opening content.screencast.com/users/Orphydian/folders/3ds%20max/media/…
-
Admin about 9 yearsawesome it worked! Just out of curiosity: why explorer exe. It opens the page in Chrome afterall. How can I open it in Internet explorer? And I replaced the "test.html" with the name of my html page. I hope thats the right way to do. Again thnaks!
-
yushulx about 9 yearsexplorer.exe will launch the default Web browser. If you want to open the page in Internet explorer, you just need to make it default: IE > Internet Options > Programs > Make default. @Orphydian
-
Dirk Bäumer about 9 yearsYou can even use variables if you have more than one HTML file. You can do: "args": ["{$file}"] to pass the current open file. See also code.visualstudio.com/Docs/tasks#_variables-in-tasksjson
-
Admin about 9 yearsI have another HTML file named "second_page.html". How should be that "args"line in this case? Do I need to keep the previous "args" line too?
-
Preetham Reddy about 9 yearsHow do I do it in Mac? There are no exe files. I would like to open the webpage in chrome on Mac
-
vallentin over 8 yearsIf someone is getting "No task runner configured", closing and re-opening Visual Studio Code worked for me!
-
johny why over 8 yearsdo you "change" parameters in task runner? or ADD your code to task runner? thx
-
johny why over 8 yearsjust getting "Failed to launch external program chrome {$file}. spawn chrome ENOENT"
-
klewis over 8 yearsThis process worked for me, but why do we have to hard-code "test.html" into this process? can we not preview any .html file in this directory through by simply opening it up and pressing ctrl+shift+b? I have multiple html files I would like to preview at any given time in my folder...
-
Kirill Osenkov almost 8 yearsI had to run
npm install -g gulp
,npm install -g gulp-webserver
and add a NODE_PATH environment variable that points to my AppData\npm\node_modules. Then I was able to run the webserver task, however I get a 404 when the browser starts. Any idea what I'm missing? -
Kirill Osenkov almost 8 yearsNever mind, just had to change the 'app' in your example to '.' (so it serves from the current directory).
-
yu yang Jian almost 8 yearsTo Configure Task in a new folder: Select the Tasks: Configure Task Runner command and you will see a list of task runner templates. Select Others to create a task which runs an external command. . . . You should now see a tasks.json file in your workspace .vscode folder with the following content: . . . by code.visualstudio.com/Docs/editor/tasks
-
jla over 7 yearsWhat version of Visual Studio Code are you using? Those commands aren't working in my just-updated 1.8.1. Ctrl+F1 does nothing and I don't have a View in Browser option in the command palette. Maybe you have something beyond the default installed or extra tasks in your tasks.json?
-
PersyJack over 7 yearsI have the same version but I realized I installed an extension for it. It is: marketplace.visualstudio.com/…
-
Friis1978 over 7 yearsOne comment to the answer: If you want to run an html file in yor browser, which will automatic reload on changes, your gulpfile.js should look like this, with a '.' instead of the 'app'. Code = var gulp = require('gulp'), webserver = require('gulp-webserver'); gulp.task('webserver', function () { gulp.src('.') .pipe(webserver({ fallback: 'index.html', livereload: true, open: true })); });
-
Gwater17 over 7 yearsWhy is the shortcut cmd + shift + b? I want to add similar shortcuts for firefox and safari but I'm not clear how I would be able to configure the keyboard shortcuts?
-
ankush981 over 7 yearsThanks! I'm a Linux user and was feeling lost. I'd like to add that one needs to press
Ctrl + Shift + b
to launch it in the browser. For me, the mnemonic was "b = browser". :-) -
guest-418 about 7 years@MJGwater in 1.10 you can assign your shortcut by editing
keybindings.json
and inserting there entry like{ "key": "<shortcut>", "command": "workbench.action.tasks.runTask", "args":"<task name>" }
, By default there are shortcuts for build('cmd+shift+b') and test('cmd+shift+t') tasks. -
Jon Crowell about 7 yearsThat extension gets horrible reviews.
-
klewis about 7 yearsThis is awesome. Thanks for explaining this as a valuable option. I also had apply a little bit of both Krill and Friis's answers to get this working. But it does!
-
Jude Niroshan about 7 yearsThis doesn't automatically refresh the browser and this is not what OP is looking for
-
Ryan B about 7 yearsI was copying only the
tasks
part. the"args":["/C"]
is what makes this work. Out of curiosity, what does this do? -
Admin almost 7 yearsI was getting "Cannot GET /" because I copypasted gulpfile.js without configuring the line
gulp.src('app')
so that it actually pointed to my source (which wasn't/app
but '.'). Works perfect. Thanks! -
M. Sundstrom about 6 yearsThis extension also has a web add-on to provide automatic reload functionality to dynamic pages.
-
user5389726598465 about 6 yearsThe question is about how to view in a browser.
-
lonstar about 6 yearsConfigure Task Runner is no longer present in VSC 1.24.0 - Control-Shift-P returns no results for that string.
-
scottdavidwalker almost 6 yearsConfigure Task Runner has changed to "Tasks: Configure Task"
-
Adil Saju over 5 years@M.Sundstrom can you give me the name/link of that add-on please?
-
Dinoel Vokiniv over 5 yearsStill very useful, and especially completely configuration free!
-
Yasser Jarouf over 5 yearsThis is not recommended for complex pages maybe good for new coders
-
M. Sundstrom over 5 yearsDo you mean the browser extension or the vs code extension @YasserAkram?
-
Yasser Jarouf over 5 yearsWell, both maybe. I never had a good experience with it. Even the Atom one. Currently I'm much better fine with Brackets.IO for basic coding and templating @M.Sundstrom
-
Bruno L. over 5 yearsIt works but seems deprecated, if you have an updated version, thank you
-
Sheece Gardazi over 5 yearstested on win 7, works like a charm. basically creates a local server.
-
JinSnow about 5 yearsbut it seems you can't preview the unsaved active file right? (Running it, doesn't preview the html, but shows on the browser the folder hierarchy)
-
JinSnow about 5 yearsPressing f5 on an unsaved HTML file send an
'${file}' can not be resolved. Please open an editor.[Open launch.json]
-
questionbank almost 5 yearsWhat about PHP extension?
-
Rodney over 4 years1.) Right-click 2.) Click "View In Browser". Oh wait, that was just a dream.
-
A__ over 4 years@Rodney marketplace.visualstudio.com/…
-
Timo over 3 yearsdoes it show changes in Javascript on save live in browser?
-
Timo over 3 yearsWorks great in Windows.
-
Timo over 3 yearsI cannot find the default keybindings.
-
Timo over 3 yearsCan you leave the version entry here in the tasks.json?
-
Timo about 3 yearsThe shortcut
ctrl+1
overwrites the defaultctrl+1
intelligently, opening a browser when inhtml
in explorer, otherwise it sets the cursor in the currenteditor
. -
Matt Croak almost 3 yearsWorks for mac too. You just need to use
Cmd
+Shift
+X
and then the rest is the same. -
Timo almost 3 yearsWith
F1
tasks: configure task
I getselect a task to configure
with . Which one to take? Maybecreate task from template
. -
Timo almost 3 yearsPlaying with
tasks.json
is no fun, be told and keep your beloved at home. -
sourcream about 2 years@JinSnow feature is on beta
-
Soren about 2 yearsDoes that work when the files sit on a remote server ?
-
Ivan Juliant about 2 yearsthank you so much , this is so much helpful to running html only , auto reload just only click go live :)