How to write properly a groovy unit test with jUnit?
14,877
length
is a (non-static) property of the sample
class. Because it's non-static, you need to evaluate it against a sample
instance, e.g.
class sampleTest extends GroovyTestCase {
@Test
public void testLength() {
def result = new sample().length([2, 3, 8, 9, 0, 1, 5, 7])
assertEquals 8, result
}
}
Also, you should try to use the assertion methods provided by GroovyTestCase
such as assertEquals
, assertNotNull
, assertTrue
, rather than the assert
keyword.
You should also capitalize your class names, e.g. Sample
and SampleTest
rather than sample
and sampleTest
.
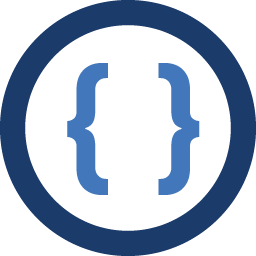
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am getting started with Groovy and I don't know how to write unit tests over my methods written on a class. For example:
One my methods on sample.groovy :
class sample { def length = { list -> list.size() } ... }
So, I have one class named sampleTest.groovy :
class sampleTest extends GroovyTestCase { @Test public void testLength() { def result = length([2, 3, 8, 9, 0, 1, 5, 7]) assert result == 8 } }
BTW, when I run this test, an error throws to me:
groovy.lang.MissingMethodException: No signature of method: sampleTest.length() is applicable for argument types: (java.util.ArrayList) values: [[2, 3, 8, 9, 0, 1, 5, 7]] at org.codehaus.groovy.runtime.ScriptBytecodeAdapter.unwrap(ScriptBytecodeAdapter.java:55) at org.codehaus.groovy.runtime.callsite.PogoMetaClassSite.callCurrent(PogoMetaClassSite.java:78) at org.codehaus.groovy.runtime.callsite.CallSiteArray.defaultCallCurrent(CallSiteArray.java:49) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.callCurrent(AbstractCallSite.java:133) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.callCurrent(AbstractCallSite.java:141) ...
-
Admin over 9 yearsThanks a lot, your answer was very useful for me.
-
TheOperator over 8 yearsThe official Groovy homepage advertises the use of power assertions (
assert
keyword) for testing.