How would I create a reset button for my program relating with the following code?
12,393
Solution 1
By calling main()
again, you are simply creating another instance of the GUI. What you should do instead is (if I understand correctly), reset the values of the currently existing GUI. You can use the set()
method of the GUI objects.
Does
def reset_values():
kiloMent.set(0)
mileMent.set(0)
reset = Button(text="Reset Values!", command=reset_values).place(x=10, y=165)
do the trick?
Looking at your code more thoroughly, however, there are some other problems there, as well. To start with, I would suggest not creating a Label
everytime the user tries to convert a value.
Solution 2
This code should work:
from tkinter import *
def main():
def closeWin():
myGui.destroy() # Close Window Function
def kiloFunc():
finalKilo.set(kiloMent.get() * 0.62) # Kilometers to Miles Fuction
def mileFunc():
finalMile.set(mileMent.get() // 0.62) # Miles to Kilometers Function
def clearFunc():
kiloMent.set("0")
mileMent.set("0")
finalKilo.set("")
finalMile.set("")
myGui = Tk()
kiloMent = IntVar()
mileMent = IntVar()
finalKilo = StringVar()
finalMile = StringVar()
myGui.title("Distance Converter")
myGui.geometry("450x200+500+200")
myLabel = Label(text="Welcome! Please enter your value then choose your option:", fg="blue", justify='center')
myLabel.pack()
kiloEntry = Entry(myGui, textvariable=kiloMent, justify='center')
kiloEntry.pack()
kilo2milesButton = Button(text="Kilometers to Miles!", command=kiloFunc)
kilo2milesButton.pack()
mileEntry = Entry(myGui, textvariable=mileMent, justify='center')
mileEntry.place(x=130, y=105)
miles2kiloButton = Button(text="Miles to Kilometers!", command=mileFunc)
miles2kiloButton.place(x=150, y=135)
kiloLabel = Label(textvariable=finalKilo, fg='red', justify='center')
kiloLabel.place(x=200, y=80)
mileLabel = Label(textvariable=finalMile, fg='red', justify='center')
mileLabel.place(x=200, y=170)
reset = Button(text="Reset Values!", command=clearFunc)
reset.place(x=10, y=165)
quit = Button(text="Quit", command=closeWin)
quit.place(x=385, y=165)
myGui.mainloop()
main()
A few notes about your original code besides the ones that Chuck mentioned:
- The
math
andsys
imports were unused. - You were setting variables equal to
widget.pack()
andwidget.place()
, which are functions that returnNone
.
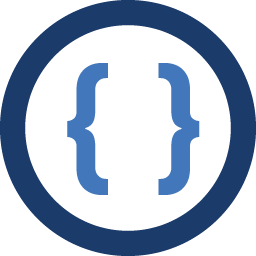
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I am trying to add a reset button but I can't seem to get it to work. I created a main in order to refer back to it when the button is pressed but no luck. Any ideas?
import sys from tkinter import * import math def main(): def closeWin(): myGui.destroy() #Close Window Function def kiloFunc(): myText = kiloMent.get() #Kilometers to Miles Fuction convert = 0.62 miles = myText * convert finalKilo = Label(text = miles,fg='red',justify='center').place(x=200,y=80) def mileFunc(): myText2 = mileMent.get() #Miles to Kilometers Function convertTwo = myText2 // 0.62 finalMile = Label(text = convertTwo, fg = 'red',justify='center').place(x=200,y=170) myGui = Tk() kiloMent = IntVar() mileMent = IntVar() myGui.title("Distance Converter") myGui.geometry("450x200+500+200") myLabel = Label(text="Welcome! Please enter your value then choose your option:",fg="blue",justify='center') myLabel.pack() kiloEntry = Entry(myGui, textvariable = kiloMent,justify='center').pack() kilo2milesButton = Button(text = "Kilometers to Miles!", command = kiloFunc).pack() mileEntry = Entry(myGui, textvariable = mileMent,justify='center').place(x=130,y=105) miles2kiloButton = Button(text = "Miles to Kilometers!", command = mileFunc).place(x=150,y=135) reset = Button(text = "Reset Values!", command = main).place(x=10,y=165) quit = Button(text="Quit", command = closeWin).place(x=385,y=165) myGui.mainloop() main()
-
Admin about 8 yearsI don't know how I didn't think of that! Thank you!
-
Admin about 8 yearsReturn none? Sorry for the stupid question I am fairly new to python. Could you explain more about that?
-
Admin about 8 yearsWhat other options do I have regarding how to display information if I am not using a label
-
tjohnson about 8 years
myLabel = Label()
is what you want to do, because it sets the value ofmyLabel
to aLabel
widget. ButmyLabel = Label().pack()
sets the value ofmyLabel
toNone
, because it is equivalent totemp = Label(); myLabel = temp.pack()
.pack()
is a function that returns the valueNone
.