How would I make a random hexdigit code generator using .join and for loops?
Solution 1
Strings can be iterated over, so my code would look like this.
import random
def gen_hex_colour_code():
return ''.join([random.choice('0123456789ABCDEF') for x in range(6)])
if __name__ == '__main__':
print gen_hex_colour_code()
results in
In [8]: 9F04A4
In [9]: C9B520
In [10]: DAF3E3
In [11]: 00A9C5
You could then put this in a separate file called for example, myutilities.py
Then in your main python file, you would use it like this:
import myutilities
print myutilities.gen_hex_colour_code()
The if __name__ == '__main__':
part will only get executed if you run the myutilities.py file directly. It will not execute when you import it from another file. This is generally where testing functions go.
Also, note that this is using the syntax for Python 2.7. In Python 3.0, one major difference is that print is a function and you would have to use print(gen_hex_colour_code()) instead. See http://docs.python.org/3.0/whatsnew/3.0.html for more info on how things are different if you are confused.
Why would I still be using Python 2.7? Many scientific python modules are still using the 2.7 variant, but for a newbie to Python, I would suggest you stick with 3.0
Solution 2
One compact way to do this is to use list comprehensions (which are a certain kind of for loop):
>>> alpha = ("0", "1", "2", "3", "4", "5", "6", "7", "8", "9",
"A", "B", "C", "D", "E", "F")
>>> ''.join([random.choice(alpha) for _ in range(6)])
'4CFDE4'
You could shorten the alphabet line by using range
and map
:
>>> alpha = map(str, range(10)) + ["A", "B", "C", "D", "E", "F"]
Or simply just use a string:
>>> alpha = "ABCDEF0123456789"
PS. Since colors are hex, why not just generate a random number and turn it to hexadecimal?
>>> hex(random.randint(0, 16777215))[2:].upper()
'FDFD4C'
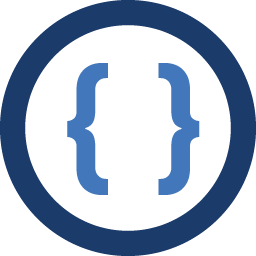
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I am new to programming and one assignment I have to do is create a random hexdigit colour code generator using for loops and .join. Is my program below even close to how you do it, or is it completely off? And, is there a way to make a random amount of numbers and letters appear within 6?
import random str = ("A","B","C","D","E","F","G","H") seq = ("1","2","3","4","5","6", "7","8","9") print '#', for i in range(0,3): letter = random.choice(str) num = random.choice(seq) print num.join(letter), print letter.join(num)