HSV OpenCv colour range
Inspired from the answer at answers.opencv link.
According to docs here
the HSV ranges like H from 0-179, S and V from 0-255, so as for your requirements for lower range and upper range example you can do for any given [h, s, v] to
[h-10, s-40, v-40]
for lower
and
[h+10, s+10, v+40]
for upper
for the yellow,green,red,blue,black,white,orange
rgb values.
Copied code from the example :
import cv2
import numpy as np
image_hsv = None # global ;(
pixel = (20,60,80) # some stupid default
# mouse callback function
def pick_color(event,x,y,flags,param):
if event == cv2.EVENT_LBUTTONDOWN:
pixel = image_hsv[y,x]
#you might want to adjust the ranges(+-10, etc):
upper = np.array([pixel[0] + 10, pixel[1] + 10, pixel[2] + 40])
lower = np.array([pixel[0] - 10, pixel[1] - 10, pixel[2] - 40])
print(pixel, lower, upper)
image_mask = cv2.inRange(image_hsv,lower,upper)
cv2.imshow("mask",image_mask)
def main():
import sys
global image_hsv, pixel # so we can use it in mouse callback
image_src = cv2.imread(sys.argv[1]) # pick.py my.png
if image_src is None:
print ("the image read is None............")
return
cv2.imshow("bgr",image_src)
## NEW ##
cv2.namedWindow('hsv')
cv2.setMouseCallback('hsv', pick_color)
# now click into the hsv img , and look at values:
image_hsv = cv2.cvtColor(image_src,cv2.COLOR_BGR2HSV)
cv2.imshow("hsv",image_hsv)
cv2.waitKey(0)
cv2.destroyAllWindows()
if __name__=='__main__':
main()
Above code is for when you want to directly select the HSV range from the image or video you are capturing, by clicking on the desired color.
If you want to predefine your ranges you can just use write simple code snippet using inbuilt python library colorsys
to convert rbg to hsv using colorsys.rgb_to_hsv
function
Note this function accepts rgb values in range of 0 to 1 only and gives hsv values also in 0 to 1 range so to use the same values you will need to normalize it for opencv
code snippet
import colorsys
'''
convert given rgb to hsv opencv format
'''
def rgb_hsv_converter(rgb):
(r,g,b) = rgb_normalizer(rgb)
hsv = colorsys.rgb_to_hsv(r,g,b)
(h,s,v) = hsv_normalizer(hsv)
upper_band = [h+10, s+40, v+40]
lower_band = [h-10, s-40, v-40]
return {
'upper_band': upper_band,
'lower_band': lower_band
}
def rgb_normalizer(rgb):
(r,g,b) = rgb
return (r/255, g/255, b/255)
def hsv_normalizer(hsv):
(h,s,v) = hsv
return (h*360, s*255, v*255)
rgb_hsv_converter((255, 165, 0))
will return
{'upper_band': [48.82352941176471, 295.0, 295.0], 'lower_band': [28.82352941176471, 215.0, 215.0]}
which is your orange hsv bands.
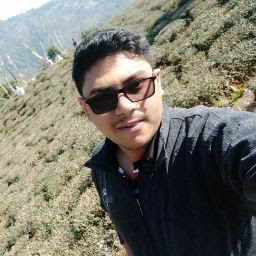
Priyom saha
I am a student and personally an adventurous guy.... Presently workng on creating 2 websites one is commercial and another a social media site
Updated on July 02, 2022Comments
-
Priyom saha almost 2 years
Can anyone please tell me a name of a website or any place from where I can get the upper and lower range of HSV of basic colours like
yellow,green,red,blue,black,white,orange
Actually I was making a bot which would at first follow black coloured line and then in the middle of the line there would be another colour given from where 3 different lines of different colour gets divided.The bot needs to decide which line to follow. For that I need the proper range of hsv colours