HTML tag <a> want to add both href and onclick working
Solution 1
You already have what you need, with a minor syntax change:
<a href="www.mysite.com" onclick="return theFunction();">Item</a>
<script type="text/javascript">
function theFunction () {
// return true or false, depending on whether you want to allow the `href` property to follow through or not
}
</script>
The default behavior of the <a>
tag's onclick
and href
properties is to execute the onclick
, then follow the href
as long as the onclick
doesn't return false
, canceling the event (or the event hasn't been prevented)
Solution 2
Use jQuery. You need to capture the click
event and then go on to the website.
$("#myHref").on('click', function() {
alert("inside onclick");
window.location = "http://www.google.com";
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<a href="#" id="myHref">Click me</a>
Solution 3
To achieve this use following html:
<a href="www.mysite.com" onclick="make(event)">Item</a>
<script>
function make(e) {
// ... your function code
// e.preventDefault(); // use this to NOT go to href site
}
</script>
Here is working example.
Solution 4
No jQuery needed.
Some people say using onclick
is bad practice...
This example uses pure browser javascript. By default, it appears that the click handler will evaluate before the navigation, so you can cancel the navigation and do your own if you wish.
<a id="myButton" href="http://google.com">Click me!</a>
<script>
window.addEventListener("load", () => {
document.querySelector("#myButton").addEventListener("click", e => {
alert("Clicked!");
// Can also cancel the event and manually navigate
// e.preventDefault();
// window.location = e.target.href;
});
});
</script>
Related videos on Youtube
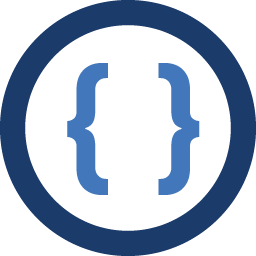
Admin
Updated on March 20, 2020Comments
-
Admin about 4 years
I'd like to ask about HTML tag
<a href="www.mysite.com" onClick="javascript.function();">Item</a>
How to make this a tag working with href and onClick? (prefer onClick running first then href)
-
Mohamed over 2 yearsCheck this Set A tag link in HTML using JavaScript
-
Menaim about 2 yearsThis one may help, It works for me : stackoverflow.com/a/7347854/14437411
-
-
Admin about 11 yearsi won't use any javascript framework. but thank you for your answer
-
Nux over 7 yearsAbove is not an actual link. It cannot be opened in a new tab and is a very bad practice. If something can be opened in a new tab (has na url) always add a meaningful
href
attribute. -
TheEquah about 7 yearsIs there also a way to do this with an element.addEventListener function?
-
yegor256 over 6 yearsDoesn't work for me, until I put
href="#"
there instead of a real URL. -
Vajira Prabuddhaka over 6 yearsI'm using laravel framework, so in my blade view I included this, It is not working, have anyone tried this with laravel?
-
DharaPPatel over 5 yearsThis solution is not working if i have any action method called in mvc controller in href. Can somebody please help ?
-
Ben over 5 yearsThis works for me in Chrome however Safari seems to run the function but not open the href...any sugestions?
-
Kamil Kiełczewski over 5 years@Ben I made test on fiddle without JS - only pure html:
<a href="http://example.com" >Item</a>
and in Chrome i see some message to allow page to run this link (alert at the end of chrome url bar). In safari in console I see warrning: [blocked] The page at fiddle.jshell.net/_display was not allowed to display insecure content from example.com - so probably this is some security issue (only on fiddle ? ) -
Corrodian about 4 yearsOnly in AngularJS