HTTP Get: Only download the header? (HEAD is not supported)
Solution 1
Instead of making a GET request, you might consider just making a HEAD request:
The HEAD method is identical to GET except that the server MUST NOT return a message-body in the response. The metainformation contained in the HTTP headers in response to a HEAD request SHOULD be identical to the information sent in response to a GET request. This method can be used for obtaining metainformation about the entity implied by the request without transferring the entity-body itself. This method is often used for testing hypertext links for validity, accessibility, and recent modification.
Solution 2
You might be able to use the Range
header in your request to specify a range of bytes to include in the response entity. Possibly something like:
Range: bytes=0-0
If it does work, you should receive back a 206 Partial Content
with the bytes specified in your Range
header present in the response entity. However, I've not tried this, and it's also not guaranteed to work:
A server MAY ignore the Range header.
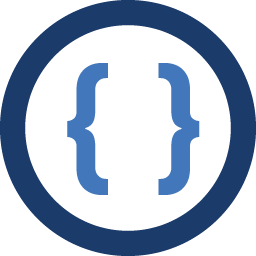
Admin
Updated on July 15, 2022Comments
-
Admin almost 2 years
In my code I use some Http Get request to download some files as a stream. I use the following code:
public String getClassName(String url) throws ClientProtocolException, IOException { HttpResponse response = sendGetRequestJsonText(url); Header[] all = response.getAllHeaders(); for (Header h : all) { System.out.println(h.getName() + ": " + h.getValue()); } Header[] headers = response.getHeaders("Content-Disposition"); InputStreamParser.convertStreamToString(response.getEntity().getContent()); String result = ""; for (Header header : headers) { result = header.getValue(); } return result.substring(result.indexOf("''") + "''".length(), result.length()).trim(); }
But this downloads the full content of the response. I want to retrieve only the http headers without the content. A HEAD request seems not to work because then i get the status 501, not implemented. How can I do that?
-
RoflcoptrException over 12 yearsI tried this, but the header looks different now :( I checked again and the status code is 501, not implemented. So my server seems not to handle such request. Are there other possibilities?
-
Rob Hruska over 12 years@Roflcoptr - That's lame. You might be able to use the
Range
header to request a 0-0 range of the entity. But I've never usedRange
headers or partial responses, so I can't really speak to that. Perhaps someone else can chime in. Also, it's possible that the server will ignore these headers, too. -
RoflcoptrException over 12 yearsFor now I found a workaround. I make a get request but as soon as I recieved the header, i call abort. Is this good practice?
-
Rob Hruska over 12 years@Roflcoptr - That sounds... sketchy. I guess I don't know off the top of my head what the consequences of that would be, but it gives me a bad vibe.
-
irreputable over 12 yearswhat's the odd that a server implements Range but not HEAD? also, Range 0-0 covers 1 byte.
-
Rob Hruska over 12 years@irreputable - Yeah, that's my thinking too; it's likely that the server won't have this available given the non-implemented
HEAD
. As for the 1-byte thing, RFE2616 (section 14.35.1) says: "If the last-byte-pos value is present, it MUST be greater than or equal to the first-byte-pos in that byte-range-spec, or the byte- range-spec is syntactically invalid." - to me, this says that a 0-byte range is valid. -
irreputable over 12 years0-0 is valid, but it means 1 byte, the 0th byte. you cannot request 0 bytes; a 206 response cannot return 0 bytes. that's indeed a little odd. although in practice, requesting 0 bytes wouldn't be useful; but not being able to do so is contrary to programmer instincts.
-
Rob Hruska over 12 years@irreputable - Reading it again, that makes sense (given that the range is inclusive). I suppose if the OP's trying to limit the number of bytes transferred, 0 is better than 1 is better than 10,000 (or the entire entity). So it might suffice as a hack to reduce the number of bytes transferred.