I want to get woocommerce reviews by product id and display it in a template
Solution 1
Woocommerce made a reviews out of regular wordpress comments.
The simple way is to get a comments of 'post_type' => 'product', that will get raw comment data. In order to display proper woocommerce review out of that you need to apply a callback function witch is 'callback' => 'woocommerce_comments'.
The whole thing is:
<?php
$args = array ('post_type' => 'product');
$comments = get_comments( $args );
wp_list_comments( array( 'callback' => 'woocommerce_comments' ), $comments);
?>
If you want to get the comment by product ID then you need to change that $args:
$args = array ('post_id' => 123);
To customize it more check out that references:
http://codex.wordpress.org/Function_Reference/wp_list_comments http://codex.wordpress.org/get_comments
The 'callback' => 'woocommerce_comments' function uses a template located in plugins/woocomerce/templates/single-product/review.php
Solution 2
To display comments (reviews) simply use:
echo comments_template();
on the product page.
For more details visit here
Solution 3
<div id="reviews">
<div id="comments">
<h2><?php
if ( get_option( 'woocommerce_enable_review_rating' ) === 'yes' && ( $count = $product->get_rating_count() ) )
printf( _n( '%s review for %s', '%s reviews for %s', $count, 'woocommerce' ), $count, get_the_title() );
else
_e( 'Reviews', 'woocommerce' );
?></h2>
<?php if ( have_comments() ) : ?>
<ol class="commentlist">
<?php wp_list_comments( apply_filters( 'woocommerce_product_review_list_args', array( 'callback' => 'woocommerce_comments' ) ) ); ?>
</ol>
<?php if ( get_comment_pages_count() > 1 && get_option( 'page_comments' ) ) :
echo '<nav class="woocommerce-pagination">';
paginate_comments_links( apply_filters( 'woocommerce_comment_pagination_args', array(
'prev_text' => '←',
'next_text' => '→',
'type' => 'list',
) ) );
echo '</nav>';
endif; ?>
<?php else : ?>
<p class="woocommerce-noreviews"><?php _e( 'There are no reviews yet.', 'woocommerce' ); ?></p>
<?php endif; ?>
</div>
<?php if ( get_option( 'woocommerce_review_rating_verification_required' ) === 'no' || wc_customer_bought_product( '', get_current_user_id(), $product->id ) ) : ?>
<div id="review_form_wrapper">
<div id="review_form">
<?php
$commenter = wp_get_current_commenter();
$comment_form = array(
'title_reply' => have_comments() ? __( 'Add a review', 'woocommerce' ) : __( 'Be the first to review', 'woocommerce' ) . ' “' . get_the_title() . '”',
'title_reply_to' => __( 'Leave a Reply to %s', 'woocommerce' ),
'comment_notes_before' => '',
'comment_notes_after' => '',
'fields' => array(
'author' => '<p class="comment-form-author">' . '<label for="author">' . __( 'Name', 'woocommerce' ) . ' <span class="required">*</span></label> ' .
'<input id="author" name="author" type="text" value="' . esc_attr( $commenter['comment_author'] ) . '" size="30" aria-required="true" /></p>',
'email' => '<p class="comment-form-email"><label for="email">' . __( 'Email', 'woocommerce' ) . ' <span class="required">*</span></label> ' .
'<input id="email" name="email" type="text" value="' . esc_attr( $commenter['comment_author_email'] ) . '" size="30" aria-required="true" /></p>',
),
'label_submit' => __( 'Submit', 'woocommerce' ),
'logged_in_as' => '',
'comment_field' => ''
);
if ( get_option( 'woocommerce_enable_review_rating' ) === 'yes' ) {
$comment_form['comment_field'] = '<p class="comment-form-rating"><label for="rating">' . __( 'Your Rating', 'woocommerce' ) .'</label><select name="rating" id="rating">
<option value="">' . __( 'Rate…', 'woocommerce' ) . '</option>
<option value="5">' . __( 'Perfect', 'woocommerce' ) . '</option>
<option value="4">' . __( 'Good', 'woocommerce' ) . '</option>
<option value="3">' . __( 'Average', 'woocommerce' ) . '</option>
<option value="2">' . __( 'Not that bad', 'woocommerce' ) . '</option>
<option value="1">' . __( 'Very Poor', 'woocommerce' ) . '</option>
</select></p>';
}
$comment_form['comment_field'] .= '<p class="comment-form-comment"><label for="comment">' . __( 'Your Review', 'woocommerce' ) . '</label><textarea id="comment" name="comment" cols="45" rows="8" aria-required="true"></textarea></p>';
comment_form( apply_filters( 'woocommerce_product_review_comment_form_args', $comment_form ) );
?>
</div>
</div>
<?php else : ?>
<p class="woocommerce-verification-required"><?php _e( 'Only logged in customers who have purchased this product may leave a review.', 'woocommerce' ); ?></p>
<?php endif; ?>
<div class="clear"></div>
Solution 4
I have been recently having the same struggle and I came up with this solution that allows you to output reviews from all products on a single page.
//Display all product reviews
if (!function_exists('display_all_reviews')) {
function display_all_reviews(){
$args = array(
'status' => 'approve',
'type' => 'review'
);
// The Query
$comments_query = new WP_Comment_Query;
$comments = $comments_query->query( $args );
// Comment Loop
if ( $comments ) {
echo "<ol>";
foreach ( $comments as $comment ): ?>
<?php if ( $comment->comment_approved == '0' ) : ?>
<p class="meta waiting-approval-info">
<em><?php _e( 'Thanks, your review is awaiting approval', 'woocommerce' ); ?></em>
</p>
<?php endif; ?>
<li itemprop="reviews" itemscope itemtype="http://schema.org/Review" <?php comment_class(); ?> id="li-review-<?php echo $comment->comment_ID; ?>">
<div id="review-<?php echo $comment->comment_ID; ?>" class="review_container">
<div class="review-avatar">
<?php echo get_avatar( $comment->comment_author_email, $size = '50' ); ?>
</div>
<div class="review-author">
<div class="review-author-name" itemprop="author"><?php echo $comment->comment_author; ?></div>
<div class='star-rating-container'>
<div itemprop="reviewRating" itemscope itemtype="http://schema.org/Rating" class="star-rating" title="<?php echo esc_attr( get_comment_meta( $comment->comment_ID, 'rating', true ) ); ?>">
<span style="width:<?php echo get_comment_meta( $comment->comment_ID, 'rating', true )*22; ?>px"><span itemprop="ratingValue"><?php echo get_comment_meta( $comment->comment_ID, 'rating', true ); ?></span> <?php _e('out of 5', 'woocommerce'); ?></span>
<?php
$timestamp = strtotime( $comment->comment_date ); //Changing comment time to timestamp
$date = date('F d, Y', $timestamp);
?>
</div>
<em class="review-date">
<time itemprop="datePublished" datetime="<?php echo $comment->comment_date; ?>"><?php echo $date; ?></time>
</em>
</div>
</div>
<div class="clear"></div>
<div class="review-text">
<div itemprop="description" class="description">
<?php echo $comment->comment_content; ?>
</div>
<div class="clear"></div>
</div>
<div class="clear"></div>
</div>
</li>
<?php
endforeach;
echo "</ol>";
} else {
echo "This product hasn't been rated yet.";
}
}
}
Add the above function to your functions.php file. After this you can use this function on your theme wherever you would like by calling it like this:
<?php echo display_all_reviews(); ?>
I also created a tutorial here https://www.majas-lapu-izstrade.lv/get-woocommerce-customer-reviews-from-all-products-display-average-and-all-ratings-in-a-histogram-without-a-plugin/ that also includes functions to output average product rating, total count of all ratings from all products and all product review histogram.
Solution 5
Yes Sir, I've tried my level best to displays the reviews at the bottom of its default tabs, but I can't do it for many days and finally I find your method to display the particular Product's reviews. It working great. Awesome Sir Awesome.
But one thing more to display the product's reviews dynamically on single-product.php // Get the Id of product dynamically and print them (reviews) by ids.
global $product;
$id = $product->id;
echo $id.",";
$args = array ('post_type' => 'product', 'post_id' => $id);
$comments = get_comments( $args );
wp_list_comments( array( 'callback' => 'woocommerce_comments' ), $comments);
Just Paste this code in your single-product.php file see the result. It will show as you want.
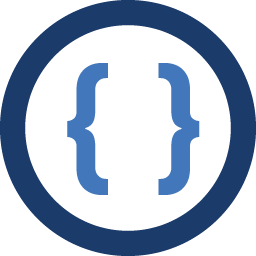
Admin
Updated on July 14, 2022Comments
-
Admin almost 2 years
I want to fetch woocommerce product reviews by product id or anything else and want to display it in a template created by me.
-
Panther almost 9 yearsadd relevant text here. External link only answers are not encourage as link mya change in future
-
Skovsgaard almost 6 yearsSimple and works great. How can I display the review form as well?