IEnumerable Concat Missing, does not contain a definition for 'Concat'
Solution 1
The compiler will give this error when the two collections are of different types T
. For example:
List<int> l1 = new List<int>();
List<string> l2 = new List<string>();
var l3 = l1.Concat(l2);
var l4 = l1.Union(l2);
The Concat
and Union
calls will result in the following compile-time errors respectively:
'List' does not contain a definition for 'Concat' and the best extension method overload 'Queryable.Concat(IQueryable, IEnumerable)' requires a receiver of type 'IQueryable'
'List' does not contain a definition for 'Union' and the best extension method overload 'Queryable.Union(IQueryable, IEnumerable)' requires a receiver of type 'IQueryable'
It is confusing because the message is misleading and VS intellisense recognizes the extension methods. The solution is that l1
and l2
must be lists of the same type T
.
Solution 2
Well this won't help you but this question is the top result when you google the error so I'm going to say what my problem was.
I had the following classes:
class Group
class Row : Group
class Column : Group
I was trying to call Concat on an IEnumerable<Row>
with an IEnumerable<Column>
to get an IEnumerable<Group>
. This doesn't work because you can't convert the columns to rows. The solution was to cast my IEnumerable<Row>
to IEnumerable<Group>
.
e.g. rows.Cast<Group>().Concat(columns)
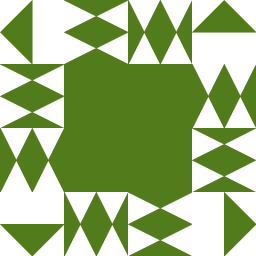
user1034912
Updated on June 25, 2022Comments
-
user1034912 6 months
I have the following Class which inherits IEnumerable
public class LinesEnumerable : IEnumerable<Point> { protected readonly IPointSeries _pointSeries; protected readonly ICoordinateCalculator<double> _xCoordinateCalculator; protected readonly ICoordinateCalculator<double> _yCoordinateCalculator; protected readonly bool _isDigitalLine; /// <summary> /// Initializes a new instance of the <see cref="LinesEnumerable" /> class. /// </summary> /// <param name="pointSeries">The point series.</param> /// <param name="xCoordinateCalculator">The x coordinate calculator.</param> /// <param name="yCoordinateCalculator">The y coordinate calculator.</param> /// <param name="isDigitalLine">if set to <c>true</c> return a digital line .</param> public LinesEnumerable(IPointSeries pointSeries, ICoordinateCalculator<double> xCoordinateCalculator, ICoordinateCalculator<double> yCoordinateCalculator, bool isDigitalLine) { _pointSeries = pointSeries; _xCoordinateCalculator = xCoordinateCalculator; _yCoordinateCalculator = yCoordinateCalculator; _isDigitalLine = isDigitalLine; } /// <summary> /// Returns an enumerator that iterates through a collection. /// </summary> /// <returns> /// An <see cref="T:System.Collections.IEnumerator" /> object that can be used to iterate through the collection. /// </returns> public virtual IEnumerator<Point> GetEnumerator() { return _isDigitalLine ? (IEnumerator<Point>)new DigitalLinesIterator(_pointSeries, _xCoordinateCalculator, _yCoordinateCalculator) : new LinesIterator(_pointSeries, _xCoordinateCalculator, _yCoordinateCalculator); } /// <summary> /// Returns an enumerator that iterates through a collection. /// </summary> /// <returns> /// An <see cref="T:System.Collections.IEnumerator" /> object that can be used to iterate through the collection. /// </returns> IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } }
However, when I try to do the following:
linesEnumerable = linesEnumerable.Concat(new[] { new Point(viewportWidth, lastYCoordinate) });
it says 'System.Collections.IEnumerable' does not contain a definition for 'Concat' and the best extension method overload 'System.Linq.Queryable.Concat(System.Linq.IQueryable, System.Collections.Generic.IEnumerable)' has some invalid arguments
I already have System.Linq namespace added
Anybody know why this is happening?
-
p4r1 almost 4 yearsThis solution inspired me to look at the types of my collections to ensure they even can be concat-ed. While they are the same type, I was using an implicitly typed array with an explicitly typed array. Changing the implicit type to a explicit type fixed the error. So
(new byte[] { 0x00 }).Concat(new[] { 0x00 });
changes to(new byte[] { 0x00 }).Concat(new byte[] { 0x00 });
-
palswim over 2 yearsAnd I find that most often, I am trying to
Concat
anIEnumerable<T>
and anIEnumerable<IEnumerable<T>>
object. I have usually usedSelect
where I have meantSelectMany
.