If Else Statements with Java using JOptionPane
Solution 1
if (answer == "yes")
When comparing String
objects, you need to use the equals
method.
Example
if(answer.equals("yes")) {
// Do code.
}
NOTE: As advised on the comments, it is safer to structure the if
statement as follows:
if("yes".equals(answer)) {
// Do code.
}
This will not through a NullPointerException
is answer
is equal to null
.
Explanation
In Java, when using the ==
operator to compare subclasses of type Object
, it will check if those two objects are references to the same object. They do not check the value of that object. Because you're comparing value, you need to use the equals
method.
How it applies to your code
Well, let's look at it. You get a String
type from the response of the InputDialog
. So this is one String
. You then attempt to compare it with "yes"
. This is, what's called a String literal
, so this means an object is created there two, so two different objects.
Remember in the case of objects, you're comparing the reference types; not the object value, so that's why it always resulted in false
.
Solution 2
You must compare Strings with .equals(...)
if("yes".equals(answer))
Using ==
will compare references where as .equals(...)
compares the values.
When you want to compare Objects (classes you make or that are a part of Java such as String
), you should use .equals(...)
(unless you intentionally want to compare references). When you compare primitive data types (such as int
, double
, char
, etc.), you should use ==
.
Solution 3
You are experiencing a common issue regarding String comparisons in Java. Comparing Strings with '==' will not do what you are expecting - instead, use the '.equals()' in the String class:
if (answer.equals("yes"))
You should generally make sure you check for null as well, since performing .equals() on a null variable would cause a crash. In general, you really want your conditional to look like this:
if (answer != null && answer.equals("yes"))
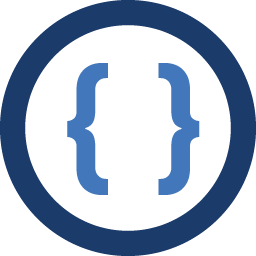
Admin
Updated on January 30, 2020Comments
-
Admin about 4 years
I am new to to programming - and am learning Java. I am trying to work an if/else statement using JOptionPane. Everything works okay, except the statements. I only get the statement "Stop and get gas!" no matter what the answer entered (yes or no).
Any help would be appreciated!
//This program determine if I have to stop for gas on the way in to work. import javax.swing.JOptionPane; import java.util.*; public class GasIndicator { public static void main(String[] args) { String answer; String outputStr1; String outputStr2; answer = JOptionPane.showInputDialog ( "Do you have at least an eighth tank of gas? yes or no " ); outputStr1 = "You can drive straight to work"; outputStr2 = "Stop and get gas!"; if (answer == "yes") JOptionPane.showMessageDialog(null, outputStr1, "Gas", JOptionPane.INFORMATION_MESSAGE); else JOptionPane.showMessageDialog(null, outputStr2, "Gas", JOptionPane.INFORMATION_MESSAGE); System.exit(0); } }
-
MadProgrammer about 10 yearsIt might be better to use "yes".equals(answer) as JOptionPane can return null
-
Catalin Pol about 10 yearsIf you use Yoda conditions (placing the constant first, so you have a non-null object reference to compare to) you can usually avoid the null check. Although it may sound a little weird, it has some advantages (see wiki link above for details).
-
Southpaw Hare about 10 yearsIt's true, I know about and agree with this methodology! I just didn't want to bring it up here since it's a fairly deep topic on its own.