Illegal call of non-static member function
Solution 1
You need to instanciate your main class and call StringToList as member:
main* m = new main;
main::node *head = m->StringToList("123");
...
delete m;
Solution 2
You will have to declare StringToList
as static
for this to work:
static node* StringToList(string number);
In this line:
main::node *head = main::StringToList("123");
You are trying to call StringToList
without having first created an object of type main
. Since it is a non-static member-function, this does not work. You'd have to do it like this:
main foo;
main::node *head foo.StringToList("123");
Which does not really make sense for your usecase, though.
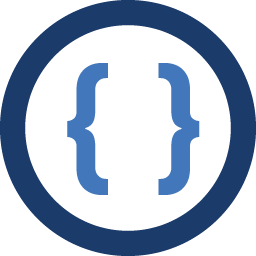
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I wish to make a calculator that can calculate numbers of almost any length.
The first function that I needed is one that converts a string into a linked list and then returns a pointer to the head of the list.
However, when compiling I am faced with an error: error C2352: 'main::StringToList' : illegal call of non-static member. Line: 7;
I provide you with my main.cpp and main.h files.
Thanks for any
main.cpp
#include "main.h" int main() { main::node *head = main::StringToList("123"); main::node *temp = new main::node; temp = head; while (temp->next != NULL) { cout << temp->data; temp = temp->next; } std::cout << "\nThe program has completed successfully\n\n"; system("PAUSE"); return 0; } main::node * StringToList(string number) { int loopTimes = number.length() - 1; int looper = 0; int *i = new int; i = &looper; main::node *temp = new main::node; main::node *head; head = temp; for ( i = &loopTimes ; *i >= 0; *i = *i - 1) { temp->data = number[*i] - 48; main::node *temp2 = new main::node; temp->next = temp2; temp = temp2; } temp->next = NULL; return head; }
main.h
#ifndef MAIN_H #define MAIN_H #include <iostream> #include <string> using namespace std; class main { public: typedef struct node { int data; node *next; }; node* StringToList (string number); }; #endif
-
Bartek Banachewicz over 11 yearsIt will work, but shouldn't the OP create a constructor instead?
-
Björn Pollex over 11 yearsWell, the OPs code is a bit problematic. For classical linked list it makes sense to have a
node
-class and a separate function to construct the list. If you would do it in the constructor of thenode
-class, you'd get a recursive constructor-call, which might be confusing. -
Mike Seymour over 11 years@Anonymous: Of course it can. If there were any non-static members then it couldn't access those, but there aren't any. (Of course, that makes the existence of the class itself rather pointless, but criticising the design is somewhat off-topic).
-
David Schwartz over 11 yearsIt makes more sense to make
StringToList
a static member function rather than creating an instance of a class. -
Björn Pollex over 11 years@Anonymous:
node
is a public nested type, and therefore can be accessed from any non-member function. -
Mike Seymour over 11 yearsAnd it makes even more sense to get rid of the class and put the function in a namespace, unless there's some non-static data, virtual functions, or whatever that were omitted from the question.
-
Mike Seymour over 11 yearsEven if it does make sense for the function to be non-static (which it probably doesn't), there's no reason to create the object with
new
, and definitely no reason to leak it like that. -
perilbrain over 11 years@DavidSchwartz:- Was thinking to write the same, but industrial C# effect just confused me.So thought better Pollex can confirm.
-
Andreas Fester over 11 yearsI definitely agree to that - there are a lot more problems with the source code shown above, be it variable naming, indentation or whatsoever, but the primary reason for the compiler error was calling a method as a static, so you either have to define it as static or call it as a member :-) (and of course clean up afterwards)
-
Mike Seymour over 11 yearsIn which case, create a temporary or automatic object. There's absolutely no reason for dynamic allocation here, even if you do remember to clean up. (Although a temporary would be problematic, since
main()
would be interpreted as a function call).