Image encryption and decryption using Pycrypto
It is just the same as encrypting or decrypting text.
Example
First import some modules:
from Crypto.Cipher import AES
from Crypto import Random
After that, let us generate a key and an initialisation vector.
key = Random.new().read(AES.block_size)
iv = Random.new().read(AES.block_size)
Encryption
Now the code below loads an input file input.jpg
and encrypts it, and afterwards it saves the encrypted data on a file encrypted.enc
. In this example, the AES block cipher is used with the CFB mode of operation.
input_file = open("input.jpg")
input_data = input_file.read()
input_file.close()
cfb_cipher = AES.new(key, AES.MODE_CFB, iv)
enc_data = cfb_cipher.encrypt(input_data)
enc_file = open("encrypted.enc", "w")
enc_file.write(enc_data)
enc_file.close()
Decryption
Finally, the code below loads the encrypted file encrypted.enc
and decrypts it, and afterwards it saves the decrypted data on a file output.jpg
.
enc_file2 = open("encrypted.enc")
enc_data2 = enc_file2.read()
enc_file2.close()
cfb_decipher = AES.new(key, AES.MODE_CFB, iv)
plain_data = cfb_decipher.decrypt(enc_data2)
output_file = open("output.jpg", "w")
output_file.write(plain_data)
output_file.close()
Note
For simplicity, the encryption and the decryption have been done in the same Python session, so the variables key
and iv
have been reused immediately, and to test this solution you have to do encryption and decryption in the same Python session. Of course, if you want to decrypt later in a separate session you will need to save key
and iv
and to reload them when you need to decrypt.
Testing the solution
Now you can open the output.jpg
file and you should see an image which is identical to the one in input.jpg
.
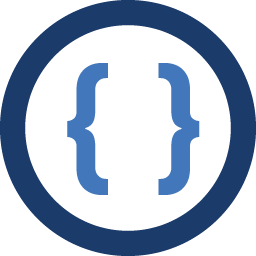
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
How can I encrypt images using the Pycrypto python library? I found some text encrypting examples on internet, but I didn't find the same with images. Can anyone help me?
-
Siddharth Choudhary almost 5 yearsWell I am trying to do something similar but am not able to convert the image to byte data and not being able to even read it through your code because it gets error of 'utf-8' codec can't decode byte 0xff in position 0: invalid start byte. Can you give a glimpse on that
-
Kubuntuer82 almost 5 yearsIs the image stored in a file?
-
Siddharth Choudhary almost 5 yearsNope, it's just an image. I actually had a look and have figured out a way to do it and it is totally different from the way you are doing it. I will soon post the answer here.
-
Kubuntuer82 almost 5 yearsPerfect @SiddharthChoudhary, well done! I look forward to seeing it :)
-
Siddharth Choudhary almost 5 yearsI can't answer this question anymore because people have closed it.
-
user311790 about 4 yearsThe input of the image in your code is giving an error
-
Kubuntuer82 about 4 years@user311790 what kind of error? It works perfectly on my machine and with my interpreter. Is your image named
input.jpg
? What Python version are you using (2 or 3)? Is PyCrypto installed in your machine? -
user311790 about 4 yearsPython 2.7.15, with pycryptodome installed, the code is working but the output image is not opening.
-
Kubuntuer82 about 4 yearsRemember that in the answer, by output file I mean
output.jpg
and notencrypted.enc
... fileoutput.jpg
is unencrypted and it is just generated to test the correctness of the encryption, it should open and should be identical toinput.jpg
(I also tested them using thediff
tool). Fileencrypted.enc
cannot be opened because it is encrypted, so if you consider that as the output file, then it's expected that it cannot be opened.