import browserHistory from react-router
16,246
As I understand your main intention is to change browser URL in the right way, not actually importing a browserHistory
For react-router
v4:
You should benefit here from HOC withRouter
. Apply it in next manner, and this will pass into your component some additional props that will allow navigation over browser history
import { withRouter } from "react-router-dom";
class Home extends Component {
home = () => {
// - you can now access this.props.history for navigation
this.props.history.push("/home");
};
render() {
return (
<button onClick={this.home} className="btn btn-outline-light">
Logout
</button>;
)
}
const HomeWitRouter = withRouter(Home);
And with react-router v5 and React Hooks this may look like:
import { useHistory } from "react-router-dom";
export const Home = () => {
const history = useHistory();
const goHome = () => {
history.push("/home");
};
return (
<button onClick={goHome} className="btn btn-outline-light">
Logout
</button>;
)
}
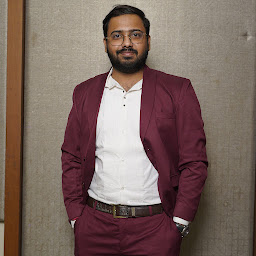
Author by
Irfan Shaikh
An idiot admires complexity, A genius admires simplicity. ⏳
Updated on June 04, 2022Comments
-
Irfan Shaikh almost 2 years
i'm trying to import browserHistory but i get error something like this.
./src/App.js Attempted import error: 'browserHistory' is not exported from 'react- router'.
And My Code is:
import React, { Component } from "react"; import { browserHistory } from "react-router"; import App from "./App"; class Home extends Component { home = () => { browserHistory.push("/home"); }; state = {}; render() { return <button onClick={this.home} className="btn btn-outline-light"> Logout </button>; }
}
-
Andrew Li over 5 yearsWhich version of React Router? In v4, individual histories were removed and replaced with routers, ie
BrowserRouter
from"react-router-dom"
-
Ionut over 5 yearsThis may explain everything github.com/ReactTraining/react-router/issues/5263
-
Andrew Li over 5 yearsPossible duplicate of Where's hashHistory in React Router v4?
-
Ionut over 5 yearsPossible duplicate of React router not showing browser history
-
-
Irfan Shaikh over 5 yearsi am trying to redirect from one page to another and when i googlr it i found this one browserHistory
-
Irfan Shaikh over 5 yearsWhy you are using this HomWitRouter it gives me warning like this is defined but it's value never used.
-
Shevchenko Viktor over 5 yearsYou need to export HomeWithRouter component and use it. Or use it in this file.