In angular 2 how to preserve query params and add additional query params to route
Solution 1
In Angular 4+, preserveQueryParams
have been deprecated in favor of queryParamsHandling
. The options are either 'merge'
or 'preserve'
.
In-code example (used in NavigationExtras
):
this.router.navigate(['somewhere'], { queryParamsHandling: "preserve" });
In-template example:
<a [routerLink]="['somewhere']" queryParamsHandling="merge">
Solution 2
It just works this way unfortunately:
const q = preserveQueryParams ? this.currentUrlTree.queryParams : queryParams;
You could try to add custom directive like this:
@Directive({selector: 'a[routerLink][merge]'})
export class RouterLinkDirective implements OnInit
{
@Input()
queryParams: {[k: string]: any};
@Input()
preserveQueryParams: boolean;
constructor(private link:RouterLinkWithHref, private route: ActivatedRoute )
{
}
public ngOnInit():void
{
this.link.queryParams = Object.assign(Object.assign({}, this.route.snapshot.queryParams), this.link.queryParams);
console.debug(this.link.queryParams);
}
}
<a [routerLink]="['/cars']" merge [queryParams]="{model: 'renault'}">Click</a>
Update: See DarkNeuron answer below.
Solution 3
There is an open issue and also already a pull request to make preserveQueryParams
a router setting instead of a per navigation setting
- https://github.com/angular/angular/issues/12664
- https://github.com/angular/angular/pull/12979
- https://github.com/angular/angular/issues/13806
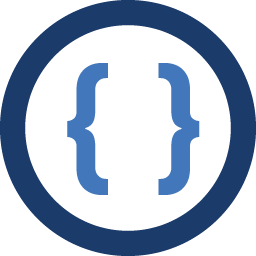
Admin
Updated on June 02, 2022Comments
-
Admin almost 2 years
For example I am on route
/cars?type=coupe
and I want to navigate to the same endpoint with additional query params (but keeping existing one). I am trying something like this<a [routerLink]="['/cars']" [queryParams]="{model: 'renault'}" preserveQueryParams>Click</a>
The initial query params are preserved (type=cars) but added ones (model=renault) are ignored. Is this expected/correct behavior or is some kind of bug? Looks like preserveQueryParams has priority over queryParams? Is there any other smooth solution?