In php when initializing a class how would one pass a variable to that class to be used in its functions?
Solution 1
I want to call a class and pass a value to it so it can be used inside that class
The concept is called "constructor".
As the other answers point out, you should use the unified constructor syntax (__construct()
) as of PHP 5. Here is an example of how this looks like:
class Foo {
function __construct($init_parameter) {
$this->some_parameter = $init_parameter;
}
}
// in code:
$foo = new Foo("some init value");
Notice - There are so-called old style constructors that you might run into in legacy code. They look like this:
class Foo {
function Foo($init_parameter) {
$this->some_parameter = $init_parameter;
}
}
This form is officially deprecated as of PHP 7 and you should no longer use it for new code.
Solution 2
In new versions of PHP (5 and up), the function __constuct is called whenever you use "new {Object}", so if you want to pass data into the object, add parameters to the construct function and then call
$obj = new Object($some, $parameters);
class Object {
function __construct($one, $two) {}
}
Named constructors are being phased out of PHP in favor of the __construct method.
Solution 3
class SomeClass
{
public $someVar;
public $otherVar;
public function __construct()
{
$arguments = func_get_args();
if(!empty($arguments))
foreach($arguments[0] as $key => $property)
if(property_exists($this, $key))
$this->{$key} = $property;
}
}
$someClass = new SomeClass(array('someVar' => 'blah', 'otherVar' => 'blahblah'));
print $someClass->someVar;
This means less maintenance in the long run.
Order of passed variables is not important anymore, (no more writing defaults like 'null': someClass(null, null, true, false))
Adding a new variable is less hassle (don't have to write the assignment in the constructor)
When you look at the instantiation of the class you'll know immediately what the passed in variables relate to:
Person(null, null, true, false)
vs
Person(array('isFat' => true, 'canRunFast' => false))
Solution 4
This is how I do mine
class MyClass {
public variable; //just declaring my variables first (becomes 5)
public variable2; //the constructor will assign values to these(becomes 6)
function __construct($x, $y) {
$this->variable = $x;
$this->variable2 = $y;
}
function add() {
$sum = $this->variable + $this->variable2
return $sum;
}
} //end of MyClass class
Create an instance and then call the function add
$myob = new MyClass(5, 6); //pass value to the construct function
echo $myob->add();
11 will be written to the page not a very useful example because you would prefer to pass value to add when you called it but this illustrates the point.
Solution 5
You can do this like that:
class SomeClass
{
var $someVar;
function SomeClass($yourValue)
{
$this->someVar = $yourValue;
}
function SomeFunction()
{
return 2 * $this->someVar;
}
}
or you can use __construct instead of SomeClass for constructor in php5.
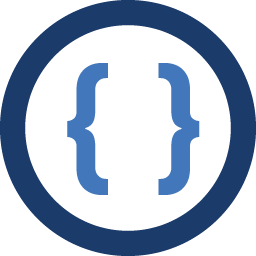
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
So here is the deal. I want to call a class and pass a value to it so it can be used inside that class in all the various functions ect. ect. How would I go about doing that?
Thanks, Sam
-
Tomalak over 15 years+1 for the deprecated warning - didn't know that. But as I see PHP it is not before version 7 that they actually do it.
-
Ciaran McNulty over 15 yearsYou probably should call the method __construct() rather than Foo, as that's the recommended behaviour in PHP5
-
Tomalak over 15 yearsHm... But did the OP mention the PHP version he uses?
-
troelskn over 15 yearsphp 4 was discontinued over a year ago.
-
Felix Kling about 13 yearsPassing arguments to a constructor is not wrong. Especially if these parameters are necessary for the working of the object. Of course one should not pass 20 parameters to a constructor...
-
giorgio over 12 years-1 as you should use __construct(), see @CiaranMcNulty 's post
-
Faizan over 11 yearsBut what if my "some init value" is in a variable called $name. How would I pass the variable in the argument of the constructor?
-
Huey over 10 yearsShouldn't it be
public $variable
&public $variable2
instead? -
spitfire over 9 yearsGreat answer. Hoping that PHP implements this RFC eventually: wiki.php.net/rfc/named_params
-
Shafizadeh over 8 yearsFor more clarification, you can define a variable in the class like this:
public $some_parameter;
. Also there is a point, the name of class should be the same with the name of method? -
Tomalak over 8 years@Sajad Yes, for "old style" constructors the name must match the name of the class. But read the first paragraph of my answer again, please.
-
pcarvalho about 8 yearstook 7 years, but they did it
-
Gregory almost 8 yearsTaking that further I would say it should be
private $variable; private $variable2;
. They should only be public if you want to allow direct access outside the class' functions, e.g.echo $myobj->variable;
-
Uriahs Victor almost 6 yearsWhat @Gregory said
-
kapitan about 2 yearsexactly what I needed, worked perfectly on my end. thank you!
[+1]