In Python, how do you convert 8-bit binary numbers into their ASCII characters?
You need to first convert it to an integer, and then you can use chr()
to get the ascii character coresponding to the value:
>>> binary_string = '01110111'
>>> int(binary_string, 2)
119
>>> chr(int(binary_string, 2))
'w'
Related videos on Youtube
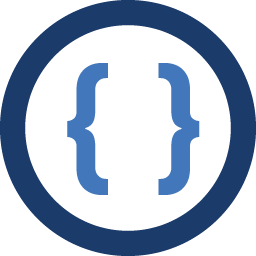
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm attempting to extract a hidden message from the blue pixels of a picture such that the if the blue value is even, it represents a 0 in the binary string, and if the blue value is odd, it represents a 1 in the binary string. The 8-bit binary strings each represent a character, and together, the characters become a hidden message.
I've broken up the whole binary string into 8-bit binary substrings, but how should I go about converting those "chunks" into ASCII characters? chr() and ord() and unichr() don't seem to be working. Is there a method or function that can be used to directly convert the 8-bit binary substring into its corresponding ASCII character, or do I have to go about manually converting the string?
import Image def chunks(s, n): for start in range(0, len(s), n): yield s[start:start+n] image = Image.open('image.png') imagePixels = image.load() imageWidth = image.size[0] imageHeight = image.size[1] blueValueEightString = "" for y in range(imageHeight): for x in range(imageWidth): blueValue = imagePixels[x, y][2] if blueValue%2 == 0: blueValue = 0 else: blueValue = 1 blueValueString = str(blueValue) blueValueEightString += blueValueString for chunk in chunks(blueValueEightString, 8): print chunk
-
tchrist about 12 yearsYou cannot convert 8-bit numbers into ASCII: you can only convert 7-bit numbers. ASCII does not hold 8 bits.
-
-
Karl Knechtel about 12 yearsIt would be much better to use arithmetic to convert the data from
imagePixels
into numbers ranging from 0-255 directly, rather than creating strings, joining them together and converting back into a number. -
Jarad almost 9 yearsI've seen the use of
int
with a base of2
in several examples now. Why does it make sense to use a base of 2?