In R how do I find whether an integer is divisible by a number?
Solution 1
for (x in 1:100) {
if (x%%5 == 0) {
print(x)
}
}
Solution 2
The modulo operator %%
is used to check for divisibility. Used in the expression
x %% y
, it will return 0 if y
is divisible by x
.
In order to make your code work, you should include an if
statement to evaluate to TRUE
or FALSE
and replace the y
with 0
inside the curly braces as mentioned above:
for (x in 1:100) {
if (x%%5 == 0) {
print(x)
}
}
For a more concise way to check for divisibility consider:
for (x in 1:100) {
if (!(x%%5)){
print(x)
}
}
Where !(x %% 5)
will return TRUE
for 0 and FALSE
for non-zero numbers.
Solution 3
How's this?
x <- 1:100
div_5 <- function(x) x[x %% 5 == 0]
div_5(x)
Solution 4
for (i in 1:10)
{
if (i %% 2)
{
#some code
}
}
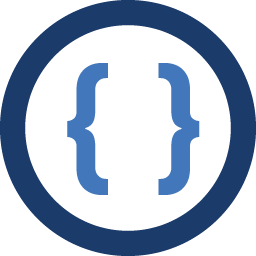
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
Sorry for the inexperience, I'm a beginning coder in R For example: If I were to make a FOR loop by chance and I have a collection of integers 1 to 100 (1:100), what would be the proper format to ensure that would print the numbers that are divisible by another number. In this case, 5 being the number divisible. I hear that using modulo would help in this instance %%
This is what I think I should have.
For (x in 1:100) { x%%5 == y } print(y)