Include xml files in maven project
Solution 1
I faced the same issue, I wanted to keep fxml & java files in the same folder (I'm using scenebuilder who needs both fxml and java files at the same place).
Here is my solution in pom.xml:
<resources>
<resource>
<directory>src/main/resources</directory>
</resource>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.fxml</include>
</includes>
</resource>
</resources>
Solution 2
If you want the xml files to be included in your JAR file, then store them in the resources folder, instead of java.
Presumably they're in src/main/java. Try moving them to src/main/resources. Anything in src/main/resources will be packaged into the JAR file along with the .class files.
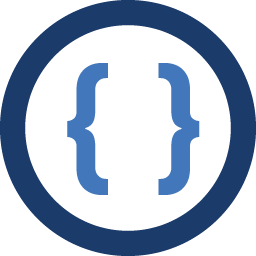
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I have a maven pom file to build from a structure like:
package1
--1.java
--2.java
--packageMetaInfo.xmlpackage2
--21.java
--22.java
--packageMetaInfo.xmlWhen I do a maven compile, the xml files don't come in the target.
maven-compiler-plugin 3.5.1 - Unless I exclude the xmls through<exclusions>
, I get an error that "Fatal error compiling: All compilation units must be of SOURCE kind ->" maven-compiler-plugin 2.0.1 - compiles but skips the xmlsIs there a way I can have the xmls included in my jar. The structure would be
x.jar
package1
--1.class
--2.class
--packageMetaInfo.xmlpackage2
--21.class
--22.class
--packageMetaInfo.xml*I understand it may not be maven standard to have xml with source files but I am working on a specific product and need to maintain this structure in both input and output.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.incent</groupId> <artifactId>Release-4.3.0.1.0.0001</artifactId> <name>AAA Custom code</name> <version>2.4.0.1</version> <properties> <ormb.cmccb.path>./Active_Repository/CMCCB</ormb.cmccb.path> <ormb.customcode.path>${ormb.cmccb.path}/data</ormb.customcode.path> <ormb.release.name>AAA-4.3.0.1.0.0001</ormb.release.name> <ormb.target.path>./target</ormb.target.path> <ormb.output.path>Release-${ormb.release.name}/Application/${ormb.release.name}/CMCCB</ormb.output.path> <ormb.serverfile.output.relpath>./target/server</ormb.serverfile.output.relpath> <build.number>SNAPSHOT</build.number> </properties> <dependencies> <dependency> <groupId>org.ow2.asm</groupId> <artifactId>asm</artifactId> <version>5.0.3</version> </dependency> <dependency> <groupId>antlr</groupId> <artifactId>antlr</artifactId> <version>2.7.7</version> <type>jar</type> </dependency> <dependency> <groupId>icu4j</groupId> <artifactId>icu4j</artifactId> <version>49.1</version> <type>jar</type> </dependency> <dependency> <groupId>dom4j</groupId> <artifactId>dom4j</artifactId> <version>1.6.1</version> <type>jar</type> </dependency> <dependency> <groupId>commons-lang</groupId> <artifactId>commons-lang</artifactId> <version>2.2</version> <type>jar</type> </dependency> <dependency> <groupId>wlfullclient</groupId> <artifactId>wlfullclient</artifactId> <version>10.3.4.0</version> <type>jar</type> </dependency> </dependencies> <build> <sourceDirectory>${ormb.customcode.path}/java</sourceDirectory> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-resources-plugin</artifactId> <version>2.6</version> <configuration> <encoding>UTF-8</encoding> </configuration> <executions> <execution> <id>copy-cm</id> <phase>install</phase> <goals> <goal>copy-resources</goal> </goals> <configuration> <outputDirectory>${ormb.customcode.path}/etc/lib</outputDirectory> <overwrite>true</overwrite> <resources> <resource> <directory>${ormb.target.path}</directory> <includes> <include>cm.jar</include> </includes> </resource> </resources> </configuration> </execution> <execution> <id>copy-mwpackage</id> <phase>install</phase> <goals> <goal>copy-resources</goal> </goals> <configuration> <outputDirectory>${ormb.serverfile.output.relpath}</outputDirectory> <overwrite>true</overwrite> <resources> <resource> <directory>${ormb.target.path}</directory> <includes> <include>Release-${ormb.release.name}.zip</include> </includes> </resource> </resources> </configuration> </execution> </executions> </plugin> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.5.1</version> <configuration> <encoding>ISO-8859-1</encoding> <source>1.7</source> <target>1.7</target> <includes> <include>**/cm/**</include> </includes> <excludes> <exclude>**/*.xml</exclude> </excludes> <resources> <resource> <directory>${ormb.customcode.path}/java</directory> <includes> <include>**/*.xml</include> </includes> </resource> </resources> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-assembly-plugin</artifactId> <version>2.6</version> <executions> <execution> <id>create-cm</id> <phase>package</phase> <goals> <goal>single</goal> </goals> <configuration> <appendAssemblyId>false</appendAssemblyId> <descriptors> <descriptor>Active_Repository/assembly/executable.xml</descriptor> </descriptors> <finalName>cm</finalName> <manifest> <addDefaultImplementationEntries>true</addDefaultImplementationEntries> </manifest> <archive> <manifestEntries> <Specification-Title>${project.name}</Specification-Title> <Specification-Version>${project.version}</Specification-Version> <Implementation-Version>${build.number}</Implementation-Version> </manifestEntries> </archive> </configuration> </execution> <execution> <id>create-distro</id> <phase>package</phase> <goals> <goal>single</goal> </goals> <configuration> <descriptors> <descriptor>Active_Repository/assembly/dist.xml</descriptor> </descriptors> <finalName>custom-action-dist</finalName> <appendAssemblyId>false</appendAssemblyId> <finalName>Release-${ormb.release.name}</finalName> </configuration> </execution> </executions> </plugin> </plugins> </build> </project>
-
EdH over 7 yearsAdditionally, if you move the xml files into src/main/resources, you won't need to exclude them from the compiler plugin