Including #includes in header file vs source file
Generally, you only want to put the minimum necessary includes into a class header file, as anyone else who uses that header will be forced to #include
all of them too. In larger projects, this leads towards slower builds, dependency issues, and all sorts of other nastiness.
Think of a header file as the public interface to your class. You don't want to saddle everyone who uses it with extra dependencies, unless they're necessary to be able to use the class.
Move anything that's only needed in the class implementation down into the source file. For other classes used in a header, only #include
their headers if you actually need to know their size or contents in the header - anything else and a forward declaration is sufficient. Most cases, you only need to #include
classes you're inheriting from, and classes whose objects are value members of your class.
This page has a good summary. (Replicated below for reference)
C++ Header File Include Patterns
Large software projects require a careful header file management even when programming in C. When developers move to C++, header file management becomes even more complex and time consuming. Here we present a few header file inclusion patterns that will simplify this chore.
Header File Inclusion Rules
Here, we discuss the basic rules of C++ header file inclusion needed to simplify header file management.
A header file should be included only when a forward declaration would not do the job.
The header file should be so designed that the order of header file inclusion is not important.
This is achieved by making sure that x.h
is the first header file in x.cpp
The header file inclusion mechanism should be tolerant to duplicate header file inclusions.
The following sections will explain these rules with the help of an example.
Header File Inclusion Example
The following example illustrates different types of dependencies. Assume a class A with code stored in a.cpp
and a.h
.
a.h
#ifndef _a_h_included_
#define _a_h_included_
#include "abase.h"
#include "b.h"
// Forward Declarations
class C;
class D;
class A : public ABase
{
B m_b;
C *m_c;
D *m_d;
public:
void SetC(C *c);
C *GetC() const;
void ModifyD(D *d);
};
#endif
a.cpp
#include "a.h"
#include "d.h"
void A::SetC(C* c)
{
m_c = c;
}
C* A::GetC() const
{
return m_c;
}
void A::ModifyD(D* d)
{
d->SetX(0);
d->SetY(0);
m_d = d;
}
File Inclusion Analysis
Lets analyze the header file inclusions, from the point of view of classes involved in this example, i.e. ABase
, A
, B
, C
and D
.
-
Class ABase:
ABase
is the base class, so the class declaration is required to complete the class declaration. The compiler needs to know the size ofABase
to determine the total size ofA
. In this caseabase.h
should be included explicitly ina.h
. -
Class B: Class
A
contains ClassB
by value , so the class declaration is required to complete the class declaration. The compiler needs to know the size of B to determine the total size ofA
. In this caseb.h
should be included explicitly ina.h
. -
Class C:
Class C
is included only as a pointer reference. The size or actual content ofC
are not important toa.h
ora.cpp
. Thus only a forward declaration has been included ina.h
. Notice thatc.h
has not been included in eithera.h
ora.cpp
. -
Class D: Class
D
is just used as a pointer reference ina.h
. Thus a forward declaration is sufficient. Buta.cpp
uses classD
in substance so it explicitly includesd.h
.
Key Points
Header files should be included only when a forward declaration will not do the job. By not including c.h
and d.h
other clients of class A
never have to worry about c.h
and d.h
unless they use class C and D by value.
a.h
has been included as the first header file in a.cpp
This will make sure that a.h
does not expect a certain header files to be included before a.h
. As a.h
has been included as the first file, successful compilation of a.cpp
will ensure that a.h
does not expect any other header file to be included before a.h
.
If this is followed for all classes, (i.e. x.cpp
always includes x.h
as the first header) there will be no dependency on header file inclusion.
a.h
includes the check on preprocessor definition of symbol _a_h_included_
. This makes it tolerant to duplicate inclusions of a.h
.
Cyclic Dependency
Cyclic dependency exists between class X
and Y
in the following example. This dependency is handled by using forward declarations.
#x.h and y.h
#
/* ====== x.h ====== */
// Forward declaration of Y for cyclic dependency
class Y;
class X
{
Y *m_y;
...
};
/* ====== y.h ====== */
// Forward declaration of X for cyclic dependency
class X;
class Y
{
X *m_x;
...
};
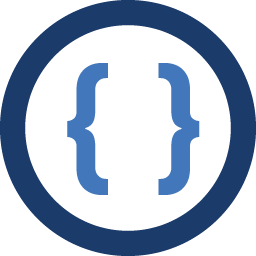
Admin
Updated on June 03, 2021Comments
-
Admin almost 3 years
I like to put all my #includes in my header file then only include my header for that source file in my source file. What is the industry standard? Are there any draw backs to my method?
-
Miguel Garcia almost 10 yearsAnd also enums you depend on since you cannot forward declare them.
-
tzaman about 9 years@Doombot thanks for the headsup, I've replicated it into the answer itself.
-
CrazyMan about 2 yearsI don't understand why including in a header and including in a source file makes a difference. Suppose I have
main.cpp
,header.h
,header.cpp
and I use#include "header.h"
inmain.cpp
. That essentially implicitly pastesheader.h
intomain.cpp
, as I understand it. Butheader.h
has no meaning withoutheader.cpp
. Isn'theader.cpp
also included with the header file? If so, why does it make a difference?