Inheritance in C++ (Parent and Child class)
At a guess I would say inheritance is not your problem, but getline
. Often the 'enter' character is left in the input buffer which means that the next input line seems to be skipped because it just reads this character. Try putting cin.get();
before your call to getline()
to flush out this extra character.
EDIT: let me refactor some of your code:
void TennisPlayer::read()
{
string tennisName;
float annualSalary;
cout << "Enter name for tennis player: " ;
cin.get(); <--read any leftover characters in the input buffer
getline(cin, tennisName); <--read in the value
set_name(tennisName); <--use parent's mutator to set the value
cout << "Enter annual salary for tennis player: ";
cin >> annualSalary;
set_annual_salary(annualSalary);
}
ADDITIONAL: The child has access to public and protected aspects of the parent class. So an alternative way to do this would be to make the member variables protected so the child class(es) can access them, but they are still hidden from external classes.
Child classes can call public member variables and functions from the parent class without scope resolution i.e. no need to specify TennisPlayer::set_name
, just set_name
will suffice.
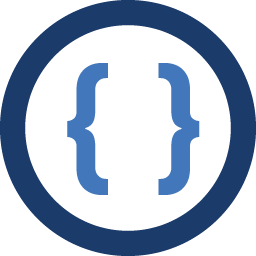
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have a parent class called Athlete and there is a child class called TennisPlayer. There are two attributes in my parent class which are name and annual salary. There is also a read method which take in user input for both classes. Here is how my parent looks like:
class Athlete { public: Athlete(); Athlete(string name, float annual_salary); virtual void read(); virtual void display(); string get_name() const; float get_annual_salary(); void set_name(string name); void set_annual_salary(float annual_salary); private: string name; float annual_salary; }; class TennisPlayer : public Athlete { public: TennisPlayer(); TennisPlayer(string name, float annual_salary, int current_world_ranking); int get_current_world_ranking(); void set_current_world_ranking(int current_world_ranking); virtual void read(); virtual void display(); private: int current_world_ranking; };
The read() method for athlete class works perfectly. But when it comes to tennisPlayer which is the child class, something went wrong, it won't take in the user input, instead it straight away ask me for the input of current world ranking. Here is my .cpp for TennisPlayer class:
void TennisPlayer::read() { cout << "Enter name for tennis player: " ; getline(cin, get_name()); cout << "Enter annual salary for tennis player: "; cin >> get_annual_salary(); cout << "Enter current world ranking: "; cin >> current_world_ranking; }
Conctructor for tennisPlayer class:
TennisPlayer::TennisPlayer(string name, float annual_salary ,int current_world_ranking) : Athlete(name , annual_salary) { this->current_world_ranking = current_world_ranking; }
Also there's a problem when reading the annual salary for tennisPlayer. I am not quite familiar with inheritance in C++. Thanks in advance.
-
Admin almost 11 yearsWhat do you mean by cin.get(). What I am trying to do is inherite the attribute from parent and override it but I have no idea how so I used accessor method instead
-
Admin almost 11 yearsBut this won't allow me to enter tennisPlayer name. It just prompt me for the input for annual salary and current world ranking. Is it something went wrong with my constructor?
-
Katstevens almost 11 yearsThis is related to my original solution, put cin.get(); before the getline(cin, tennisName);. See my amended solution
-
Katstevens almost 11 yearsBasically, getline() reads all the characters up-to-but-not-including the enter key, so you next call to getline() only reads the enter key and nothing more. It tries to assign the enter key character to tennisName then moves on to annualSalary - hence it seems like it's skipping. It's a symptom of mixing cin >> and getline().
-
Admin almost 11 yearsThanks a lot its fixed