Initialize an array of arrays in Julia
Solution 1
If you want an array of arrays as opposed to a matrix (i.e. 2-dimensional Array
):
a = Array[ [1,2], [3,4] ]
You can parameterize (specify the type of the elements) an Array
literal by putting the type in front of the []
. So here we are parameterizing the Array
literal with the Array
type. This changes the interpretation of brackets inside the literal declaration.
Solution 2
In Julia v0.5, the original syntax now produces the desired result:
julia> a = [[1, 2], [3, 4]]
2-element Array{Array{Int64,1},1}:
[1,2]
[3,4]
julia> VERSION
v"0.5.0"
Solution 3
Sean Mackesey's answer will give you something of type Array{Array{T,N},1}
(or Array{Array{Int64,N},1}
, if you put the type in front of []
). If you instead want something more strongly typed, for example a vector of vectors of Int (i.e. Array{Array{Int64,1},1}
), use the following:
a = Vector{Int}[ [1,2], [3,4] ]
Solution 4
For a general answer on constructing Arrays of type Array
:
In Julia, you can have an Array that holds other Array type objects. Consider the following examples of initializing various types of Arrays:
A = Array{Float64}(10,10) # A single Array, dimensions 10 by 10, of Float64 type objects
B = Array{Array}(10,10,10) # A 10 by 10 by 10 Array. Each element is an Array of unspecified type and dimension.
C = Array{Array{Float64}}(10) ## A length 10, one-dimensional Array. Each element is an Array of Float64 type objects but unspecified dimensions
D = Array{Array{Float64, 2}}(10) ## A length 10, one-dimensional Array. Each element of is an 2 dimensional array of Float 64 objects
Consider for instance, the differences between C and D here:
julia> C[1] = rand(3)
3-element Array{Float64,1}:
0.604771
0.985604
0.166444
julia> D[1] = rand(3)
ERROR: MethodError:
rand(3)
produces an object of type Array{Float64,1}
. Since the only specification for the elements of C
are that they be Arrays with elements of type Float64, this fits within the definition of C
. But, for D
we specified that the elements must be 2 dimensional Arrays. Thus, since rand(3)
does not produce a 2 dimensional array, we cannot use it to assign a value to a specific element of D
Specify Specific Dimensions of Arrays within an Array
Although we can specify that an Array will hold elements which are of type Array, and we can specify that, e.g. those elements should be 2-dimensional Arrays, we cannot directly specify the dimenions of those elements. E.g. we can't directly specify that we want an Array holding 10 Arrays, each of which being 5,5. We can see this from the syntax for the Array()
function used to construct an Array:
Array{T}(dims)
constructs an uninitialized dense array with element type T. dims may be a tuple or a series of integer arguments. The syntax Array(T, dims) is also available, but deprecated.
The type of an Array in Julia encompasses the number of the dimensions but not the size of those dimensions. Thus, there is no place in this syntax to specify the precise dimensions. Nevertheless, a similar effect could be achieved using an Array comprehension:
E = [Array{Float64}(5,5) for idx in 1:10]
Solution 5
For those wondering, in v0.7 this is rather similar:
Array{Array{Float64,1},2}(undef, 10,10) #creates a two-dimensional array, ten rows and ten columns where each element is an array of type Float64
Array{Array{Float64, 2},1}(undef,10) #creates a one-dimensional array of length ten, where each element is a two-dimensional array of type Float64
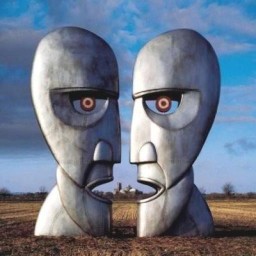
fhucho
Updated on July 01, 2020Comments
-
fhucho over 2 years
I'm trying to create an array of two arrays. However,
a = [[1, 2], [3, 4]]
doesn't do that, it actually concats the arrays. This is true in Julia:[[1, 2], [3, 4]] == [1, 2, 3, 4]
. Any idea?As a temporary workaround, I use
push!(push!(Array{Int, 1}[], a), b)
. -
Benjohn almost 8 yearsI thought
{}
was for maps – but I've tried this and see that it does indeed do as you say. There's a lot more going on here than I realise! -
David P. Sanders about 7 years
{...}
has been deprecated in Julia v0.4. -
PythonNut about 7 years@DavidP.Sanders what was that syntax replaced with?
-
David P. Sanders about 7 yearsIn the future (hopefully v0.5) it will be just what you would like to write, [ [1, 2], [3, 4] ], i.e. a vector of vectors. (In Julia v0.3 that syntax concatenated the two arrays into a single array with 4 elements. This has also been deprecated in v0.4. Concatenation is now with ";" : [ [1, 2]; [3, 4] ] gives [1, 2, 3, 4]. )
-
David P. Sanders about 7 yearsFor now, the best option is
Vector{Int}[[1, 2], [3,4]]
-
xji about 4 yearsjulia> C = Array{Array{Float64}}(10) ERROR: MethodError: no method matching Array{Array{Float64,N} where N,N} where N(::Int64) Closest candidates are: Array{Array{Float64,N} where N,N} where N(::UndefInitializer, ::Int64) where T at boot.jl:408 Array{Array{Float64,N} where N,N} where N(::UndefInitializer, ::Int64, ::Int64) where T at boot.jl:409 Array{Array{Float64,N} where N,N} where N(::UndefInitializer, ::Int64, ::Int64, ::Int64) where T at boot.jl:410 ...