Initialize struct with malloc in C
Solution 1
In addition to Michael's bug fix, I also wonder about board: it is not allocated, yet you write to it. I think it should be like:
struct game *new_game(int xsize, int ysize)
{
int black = 1;
int white = 5;
int i;
int j;
struct game *new = malloc (sizeof(struct game));
if (new == NULL) return NULL;
game->xsize= xsize;
game->ysize= ysize;
game->board= malloc(xsize*ysize*sizeof(int));
for(i=0;i<xsize;i++)
{
for(j=0;j<ysize;j++)
{
if(i<(xsize/2) && j<(ysize/2) && (i+j)%2!=0)
{
game->board[i*xsize+j] = black;
}
else if(i>(xsize/2) && j>(ysize/2) && (i+j)%2!=0)
{
game->board[i*xsize+j] = white;
}
else game->board[i*xsize+j] = 0;
}
}
return (game);
}
Note also the array indexing: the compiler doesn't know the dynamic rowsize so you have to do that yourself.
Solution 2
It happens because you only allocated space for a pointer to your struct
. What you need to do, is allocate it for the entire size of it:
struct game *new = malloc (sizeof(struct game));
Edit: Don't be mislead by the return value of malloc, as it returns a pointer to the allocated space, that's why it should be struct game *new
as it is.
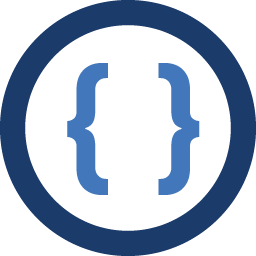
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
So I have what I think is a noob question. Sorry for that in advance, and for the grammar as english is not my primary language.
So I have to make a game of checkers. I have some struct, defined by
struct game { int **board; int xsize, ysize; struct move *moves; int cur_player; }; struct coord { int x, y; }; struct move_seq { struct move_seq *next; struct coord c_old; struct coord c_new; int piece_value; struct coord piece_taken; int old_orig; }; struct move { struct move *next; struct move_seq *seq; };
And I have to initialize a struct game wih the fonction
struct game *new_game(int xsize, int ysize)
So, here's my problem. I call, for now, new_game always with 10 and 10 values for xsize an ysize. Then I initialize the board game, which I want to assign later.
int black = 1; int white = 5; int **board; int i; int j; int k; for(i=0;i<xsize;i++) { for(j=0;j<ysize;j++) { if(i<(xsize/2) && j<(ysize/2) && (i+j)%2!=0) { board[i][j] = black; } else if(i>(xsize/2) && j>(ysize/2) && (i+j)%2!=0) { board[i][j] = white; } else board[i][j] = 0; } } struct game *new = malloc (sizeof(struct game *)); if (new == NULL) return NULL;
So, my problem is after that. I just have Segmentation Fault whatever I do with my struct new.
I tried to do assign new->xsize = xsize and the same with ysize. I do a malloc for the board and the struct move, like I learned to do, but I kept getting this error of Segmentation Fault.
So here's my real question: How to assign and initialize correctly a struct ? Do I Have to make a malloc for each of the member of struct game ? (I tried that too but without any success...)
I don't necessarily want just the answer, I'd prefer to really understand what I have to do in this case and in general, to make less mistakes in the future.
Thanks in advance for your help.
Have a good day.