Initializing C dynamic arrays
Solution 1
You need to allocate a block of memory and use it as an array as:
int *arr = malloc (sizeof (int) * n); /* n is the length of the array */
int i;
for (i=0; i<n; i++)
{
arr[i] = 0;
}
If you need to initialize the array with zeros you can also use the memset
function from C standard library (declared in string.h
).
memset (arr, 0, sizeof (int) * n);
Here 0
is the constant with which every locatoin of the array will be set. Note that the last argument is the number of bytes to be set the the constant. Because each location of the array stores an integer therefore we need to pass the total number of bytes as this parameter.
Also if you want to clear the array to zeros, then you may want to use calloc
instead of malloc
. calloc
will return the memory block after setting the allocated byte locations to zero.
After you have finished, free the memory block free (arr)
.
EDIT1
Note that if you want to assign a particular integer in locations of an integer array using memset
then it will be a problem. This is because memset
will interpret the array as a byte array and assign the byte you have given, to every byte of the array. So if you want to store say 11243 in each location then it will not be possible.
EDIT2
Also note why every time setting an int array to 0 with memset
may not work: Why does "memset(arr, -1, sizeof(arr)/sizeof(int))" not clear an integer array to -1? as pointed out by @Shafik Yaghmour
Solution 2
Instead of using
int * p;
p = {1,2,3};
we can use
int * p;
p =(int[3]){1,2,3};
Solution 3
You cannot use the syntax you have suggested. If you have a C99 compiler, though, you can do this:
int *p;
p = malloc(3 * sizeof p[0]);
memcpy(p, (int []){ 0, 1, 2 }, 3 * sizeof p[0]);
If your compiler does not support C99 compound literals, you need to use a named template to copy from:
int *p;
p = malloc(3 * sizeof p[0]);
{
static const int p_init[] = { 0, 1, 2 };
memcpy(p, p_init, 3 * sizeof p[0]);
}
Solution 4
p = {1,2,3} is wrong.
You can never use this:
int * p;
p = {1,2,3};
loop is right
int *p,i;
p = malloc(3*sizeof(int));
for(i = 0; i<3; ++i)
p[i] = i;
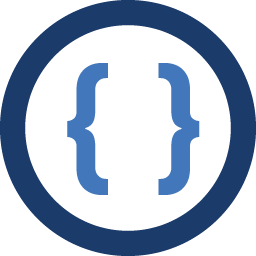
Admin
Updated on July 19, 2022Comments
-
Admin almost 2 years
How do I initialize a dynamic array allocated with malloc? Can I do this:
int *p; p = malloc(3 * sizeof(*p)); p = {0, 1, 2}; ... free(p);
Or do I need to do something like this:
int *p, x; p = malloc(3 * sizeof(*p)); for (x = 0; x < 3; x++) p[x] = 0; ... free(p);
Or is there a better way to do it?
-
Ignas2526 almost 11 yearsThis is exactly what I was looking for! Super useful if each individual element has its own value what cannot be computed in any way, so you end-up writing initialization for each element individually. One question: is it part of any C standard, or is it compiler specific?
-
user2357112 almost 8 yearsThis is not a drop-in replacement for a
malloc
-ed array! The compound literal you're using to initializep
has automatic storage duration. It will be freed automatically at the end of the block, and callingfree
on it manually is undefined behavior. You can't use this to allocate an array you're going to return, or to allocate anything that has to persist beyond the current block.