Inner Joins Query in HQL
Solution 1
Joins in HQL have a slightly different syntax.
If you've already got those associations mapped with Hibernate, the join conditions (which id
fields to do the join on) are usually handled by Hibernate itself (which already has that information defined in the mapping), so you only need to specify which attribute the association is mapped with, and do the join on it:
SELECT op.username, op.email, orders.p_id, orders.o_id, product.listed_price
FROM Orders order
INNER JOIN order.orderProcessing as op
INNER JOIN order.product as product
ORDER BY op.username
In the case you haven't got those associations mapped, you should probably use a cross join like syntax, and specify the join conditions in the WHERE
clause. Please note this could have a negative impact on efficiency depending on your schema structure and DBMS.
SELECT op.username, op.email, orders.p_id, orders.o_id, product.listed_price
FROM Orders order, OrderProcessing op, Product product
WHERE op.u_id = order.u_id AND product.p_id = orders.p_id
ORDER BY op.username
You can find more information on HQL joins in the 14.3. Associations and joins section of the Hibernate reference.
Solution 2
Joins in HQL do not work like in SQL. You can either join on an association:
select order_1.code, item.code, item.quantity
from Order order_1 inner join order_1.item item;
or use a multi-table select:
select order_1.code, item.code, item.quantity
from Order order_1, Item item where item.order=order_1;
either syntax has advantages and disadvantages, so you should choose depending on situation.
It is worth noting that there is also an implicit notation, which might make unnecessary to use the join at all:
select item.order.code, item.code, item.quantity from Item item;
Solution 3
I suspect it is because order
is a reserved word (as in order by
). Try doing FROM Orders o
instead.
I think you'll have other problems too though.
Look at what you are selecting (a bunch of random column values), and what you are expecting Hibernate to return you (List<Orders>
).
I expect you won't get back List<Orders>
because the structure of your query will force hibernate into SQL
mode instead of HQL
. In this mode, you'll just get back a List<Object[]>
where each Object[]
is a set of those four columns you are selecting.
This link explains what you can expect to get back with different queries:
http://www.laliluna.de/jpa-hibernate-guide/ch11s02.html
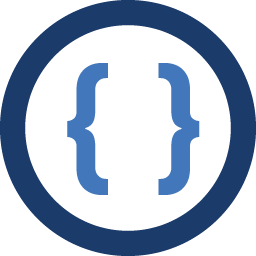
Admin
Updated on December 30, 2020Comments
-
Admin over 3 years
I'm unable to execute HQL for Inner Joins, Query is executing correct at sql but not in HQL. I don't know where i'm missing. Your help is appreciable.
***Error***: org.hibernate.hql.ast.QuerySyntaxError: unexpected token: ON near line 1, column 148 [SELECT op.username, op.email, orders.p_id, orders.o_id, product.listed_price FROM com.model.Orders orders INNER JOIN orders.OrderProcessing as op ON op.u_id = orders.u_id INNER JOIN orders.Product as product ON product.p_id = orders.p_id WHERE product.p_id = '208' ORDER BY op.username] productList = (List<Orders>) session.createQuery( "SELECT op.username, op.email, orders.p_id, orders.o_id, product.listed_price " + "FROM Orders orders " + "INNER JOIN orders.OrderProcessing as op " + "ON op.u_id = orders.u_id " + "INNER JOIN orders.Product as product " + "ON product.p_id = orders.p_id " + "WHERE product.p_id = '"+p_id +"' " + "ORDER BY op.username" ).list();
-
Admin about 11 yearsIt's still not working. SELECT op.username, op.email, orders.p_id, orders.o_id" + "FROM Orders order" + "INNER JOIN order.orderProcessing as op " + "INNER JOIN order.product as product" + "Where product.p_id="+p_id+ "ORDER BY op.username
-
Grim about 11 yearsIf that string concatenation is taken straight from the code you're using you're missing a number of spaces - before and after from Orders order, before the where and the order by clause. If that's not the problem, you might need to post the error you're getting before someone can figure out what's happening (and if possible the mapping/structure of your business objects)
-
Admin about 11 yearsorg.hibernate.hql.ast.QuerySyntaxError: unexpected token: Orders near line 1, column 60 [SELECT op.username, op.email, orders.p_id, orders.o_idFROM Orders orderINNER JOIN order.orderProcessing as op INNER JOIN order.product as productWhere product.p_id=208ORDER BY op.username]
-
Xavi López about 11 yearsThe error is clearly stating that you have
orders.p_id
in theSELECT
clause. You've got to use the same alias you gave in theFROM
clause,order
.SELECT ..., order.p_id, order.o_id
. -
Grim about 11 years@DevSingh like i pointed out previously, there are many spaces missing - before 'FROM' and before the first 'INNER JOIN', before 'Where' and before 'ORDER BY'. Also, see Mr Spoon answer below since both of its notes are true as well (order can not be used as alias - my mistake here for letting it slip in my answer - and you're not going to get back an Orders list, but some scalar values).
-
Admin about 11 years@Grim : SELECT op.username, op.email, orders.p_id, orders.o_id, product.listed_price " + "FROM Orders orders " + "INNER JOIN OrderProcessing op ON op.u_id = orders.u_id " + "INNER JOIN Product product ON product.p_id = orders.p_id WHERE product.p_id = '"+p_id + "' " + "ORDER BY op.username
-
Grim about 11 yearsThe spaces seem right - change back to joins to the form you used above ('INNER JOIN orders.orderProcessing as op' and likewise for Product)
-
Admin about 11 years@Grim SELECT op.username, op.email, orders.p_id, orders.o_id, product.listed_price " + "FROM Orders orders " + "INNER JOIN orders.OrderProcessing as op " + "ON op.u_id = orders.u_id " + "INNER JOIN orders.Product as product " + "ON product.p_id = orders.p_id " + "WHERE product.p_id = '"+p_id +"' " + "ORDER BY op.username
-
Admin about 11 yearsI'm getting an error: org.hibernate.hql.ast.QuerySyntaxError: unexpected token: ON near line 1, column 148 [SELECT op.username, op.email, orders.p_id, orders.o_id, product.listed_price FROM com.model.Orders orders INNER JOIN orders.OrderProcessing as op ON op.u_id = orders.u_id INNER JOIN orders.Product as product ON product.p_id = orders.p_id WHERE product.p_id = '208' ORDER BY op.username]