Invalid number of channels in input image
Solution 1
As error message said, the image given in input to the color conversion function has an invalid number of channels.
The point is that you are acquiring frames as single 8bit channel
Camera.set(cv::CAP_PROP_FORMAT, CV_8UC1)
and then you try to convert this frame in grayscale
cv::cvtColor(image, gray, cv::COLOR_BGR2GRAY)
You have 2 easy options to solve this issue:
- you change the camer acquisition format in order to have color informations in your frames, for example using CV_32S or CV_32F
- you skip the color conversion as you already have grayscale image, thus no need to convert it.
Take a look to this link for OpenCV color manipulation
Solution 2
You want to do color manipulation but your image has the type CV_8U1. It has to be at least a three channel image like CV_8UC3 or CV_32F. Try a different CV_Type
Camera.set(cv::CAP_PROP_FORMAT, CV_32F);
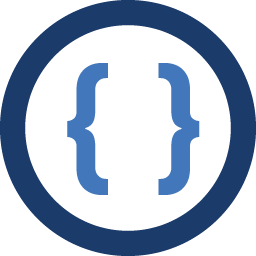
Admin
Updated on June 07, 2020Comments
-
Admin almost 4 years
I am receiving an error when running my program, I think specifically about color manipulation in the OpenCV library.
I'm trying to build a program that takes a video feed from a Raspberry Pi camera and analyze it. I want to find the brightest point in the video and calculate the distance and angle the point is at from the center of the video feed.
The project I am doing has the camera pointed at the center of a dark box, with a moving point of light.
I am using OpenCV 4.0.0 and C++ on a Raspberry Pi 3, as well as the raspicam library.
I am taking pointers from this guide, but I am using C++ and a video feed instead of Python and a static image.
raspicam::RaspiCam_Cv Camera; cv::Mat image; cv::Mat gray; int nCount=100; int nR, nC; // numRows, numCols cv::Point imgMid; Vect toCenter; // for recording brightest part of img double minVal, maxVal; cv::Point minLoc, maxLoc; Camera.set(cv::CAP_PROP_FORMAT, CV_8UC1); #ifdef DEBUG cout << "Opening camera..." << endl; if (!Camera.open()) { cerr << "Error opening the camera" << endl; return -1; } cout << "Capturing " << nCount << " frames ...." << endl; #endif for (int i=0; i< nCount; i++) { Camera.grab(); Camera.retrieve(image); nR = image.rows; nC = image.cols; imgMid.x = nC / 2; imgMid.y = nR / 2; // convert to grayscale image cv::cvtColor(image, gray, cv::COLOR_BGR2GRAY); // find x, y coord of brightest part of img cv::minMaxLoc(gray, &minVal, &maxVal, &minLoc, &maxLoc); // calculate vector to the center of the camera toCenter.first = distBtwn(imgMid.x, maxLoc.x, imgMid.y, maxLoc.y); toCenter.second = angle(imgMid.x, maxLoc.x, imgMid.y, maxLoc.y);
I expect the program to take a frame of the video feed, convert it to grayscale, find the brightest part of the frame, and finally do some calculations to find the distance to the center of the frame and angle of the point from the positive x-axis.
This is the error
I apologize for the phone camera, but I am working with somebody else in a different city, where they have the testing equipment (I am the coder) and this is what they sent me.