Invalid token '=' in class, struct, or interface member declaration c#
Solution 1
Settting a default-value for auto-implemented properties is only available from C#-version 6 and upwards. Before Version 6 you have to use the constructor and set the default-value there:
public class PlayerCharacter {
public int Health { get; private set; }
public PlayerCharacter()
{
this.Health = 100;
}
}
To enable the compiler for VS2013 you may use this approach.
Solution 2
It looks like this error happens due to version your MSBuild, old version of MSBuild can only compile C# version 4, while your code written in C# version 6 format (set default value for properties).
Example of code writing in C# version 6:
public static string HostName { get; set; } = ConfigurationManager.AppSettings["RabbitMQHostName"] ?? "";
For MSBuild to compile your code, you need to write in C# 4 style
public static string HostName { get; set; }
public SomeConstructor()
{
HostName = ConfigurationManager.AppSettings["RabbitMQHostName"] ?? "";... }
Or
public static string HostName
{
get
{
return ConfigurationManager.AppSettings["RabbitMQHostName"] ?? "";
}
}
Hope it helps
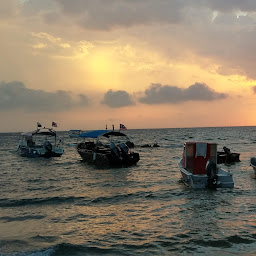
Graeme Phillips
By Day: Software Tester of over 10 years experience in both manual and automation using Selenium Webdriver (java) and BDD Cucumber & Calabash. Some TDD using basic Ruby. By Night: Drummer, Guitarist and general nerdy layabout.
Updated on July 09, 2022Comments
-
Graeme Phillips almost 2 years
this may be a simple question for people, but I can't see why this is occurring. here is my code 1st:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace GameCore { public class PlayerCharacter { public void Hit(int damage) { Health -= damage; if (Health <= 0) { IsDead = true; } } public int Health { get; private set; } = 100; public bool IsDead{ get; private set; } } }
now Visual studio is giving the Invalid token error on the assignment sign (=) (as per the title), and I can not see why. can anyone shed light on this please?
What I'm trying to do is set the int of Health to 100, and each time a character takes damage, then Health is decreased. Thanks all.
I'm using Visual Studio 2013 v12.0.40629.00 update 5
-
Graeme Phillips about 8 yearsthanks HimBromBeere - but where do I place that in the code I've copied in the main question above? sorry bit of a noob, just learning. go easy, thanks. Graeme
-
MakePeaceGreatAgain about 8 yearsDirectly into the
PlayerCharacter
-class, prefereably at its top. -
Graeme Phillips about 8 yearshi HimBromBeere - I think ive done something wrong... here is the new code, which it doesn't like at all, sorry. namespace GameCore { public class PlayerCharacter { public int Health { get; private set; } public PlayerCharacter { this.Health = 100; } public void Hit(int damage) { Health -= damage; if (Health <= 0) { IsDead = true; } } public bool IsDead{ get; private set; } } }
-
Graeme Phillips about 8 yearssorry, but totally lost now.. If I try that, then I get 7 errors instead of 1 error.
-
Graeme Phillips about 8 yearsthe Health variable only show intellisense within the Hit method.
-
Nyerguds about 8 years
public PlayerCharacter
is your class constructor, not a property. It's a function; it needs()
behind the name. -
Graeme Phillips about 8 yearsthank you very much Nyerguds... making it a function has worked! 100%
-
Jesus Campon over 5 yearsThis comment helped me more than the above, because I could build my project with no issues from my VS2017 on my computer, but I got this build failure on our CI system (TeamCity) afterwards, which was using an older version of MSBuild to build the project. So reverting the C# 6.0 property autoinitialization to an older C# syntax compatible with that MSBuild sorted out that issue - and pointed me towards the need to update our TeamCity infrastructure!
-
David C Fuchs almost 4 yearsWas pulling my hair out over this, thanks for the lead. Windows flaked out and swapped from VS 2015 to VS 2013 on me. Your answer gave me the solution, set VS 2015 as default ....